C++ Exercises: Check if a number is Keith or not
Write a C++ program to check if a number is Keith or not (with explanation).
Sample Output:
Input a number : 197
1 + 9 + 7 = 17
9 + 7 + 17 = 33
7 + 17 + 33 = 57
17 + 33 + 57 = 107
33 + 57 + 107 = 197
The given number is a Keith Number.
Sample Solution:
C++ Code:
#include<bits/stdc++.h> // Including the complete set of standard C++ libraries
using namespace std; // Using the standard namespace
// Function to count the number of digits in a number
int lenCount(int nm) {
int ctr = 0; // Counter initialized to count the digits
while (nm > 0) { // Loop to count digits
nm = nm / 10; // Remove the last digit
ctr++; // Increment the counter for each digit removed
}
return ctr; // Return the count of digits
}
// Main function
int main() {
int num1 = 0, arr1[10], num2 = 0, flg = 0, i = 0, sum = 0, kk; // Declaration of integer variables
cout << "\n\n Check whether a number is Keith or not: \n"; // Displaying purpose
cout << " Sample Keith numbers: 197, 742, 1104, 1537, 2208, 2580, 3684, 4788, 7385..\n"; // Displaying purpose
cout << " -----------------------------------------------------------------------\n"; // Displaying purpose
cout << " Input a number : "; // Prompting user for input
cin >> num1; // Taking user input
num2 = num1; // Storing the original number
// Loop to store individual digits of the number into an array in reverse order
for (i = lenCount(num2) - 1; i >= 0; i--) {
arr1[i] = num1 % 10; // Extract the last digit
num1 /= 10; // Remove the last digit
}
// Loop to check if the number is a Keith number
while (flg == 0) {
for (i = 0; i < lenCount(num2); i++)
sum += arr1[i]; // Calculate the sum of the digits in the array
// If the sum matches the original number, it's a Keith number
if (sum == num2) {
flg = 1; // Set the flag to terminate the loop
kk = 1; // Set kk to 1 indicating the number is a Keith number
}
// If the sum exceeds the original number, it's not a Keith number
if (sum > num2) {
flg = 1; // Set the flag to terminate the loop
kk = 0; // Set kk to 0 indicating the number is not a Keith number
}
// Loop to reconstruct the array by shifting elements and adding the new sum
for (i = 0; i < lenCount(num2); i++) {
cout << " " << arr1[i]; // Display the array elements
if (i != lenCount(num2) - 1) {
arr1[i] = arr1[i + 1]; // Shift elements in the array
cout << " + "; // Displaying '+' between elements
} else {
arr1[i] = sum; // Store the new sum at the last index of the array
cout << " = " << arr1[i]; // Display the sum
}
}
cout << endl; // Move to the next line
sum = 0; // Reset the sum for the next iteration
}
// Displaying whether the number is a Keith number or not based on kk value
if (kk == 1) {
cout << " The given number is a Keith Number.\n"; // Displaying output
}
if (kk == 0) {
cout << " The given number is not a Keith Number.\n"; // Displaying output
}
}
Sample Output:
Check whether a number is Keith or not: Sample Keith numbers: 197, 742, 1104, 1537, 2208, 2580, 3684, 4788, 7385.. ----------------------------------------------------------------------- Input a number : 197 1 + 9 + 7 = 17 9 + 7 + 17 = 33 7 + 17 + 33 = 57 17 + 33 + 57 = 107 33 + 57 + 107 = 197 The given number is a Keith Number.
Flowchart:
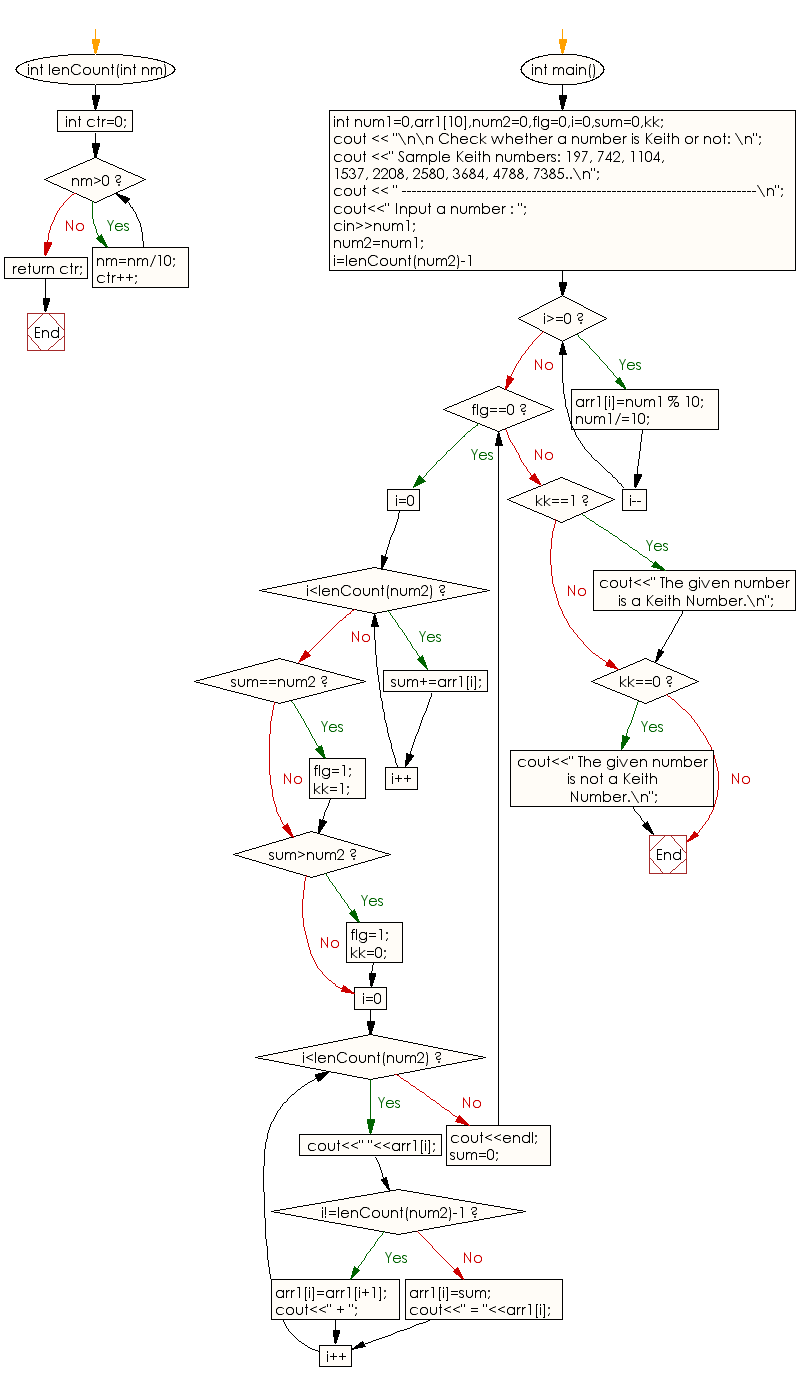
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Find Strong Numbers within a range of numbers.
Next: Create the first twenty Hamming numbers.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics