C++ Exercises: Check whether a given number is Perfect or not
4. Perfect Number Check
Write a program in C++ to check whether a given number is Perfect or not.
Visual Presentation:
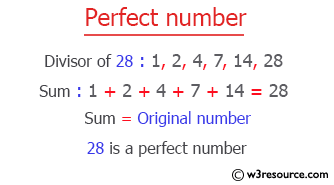
Sample Solution:
C++ Code :
# include <iostream> // Include necessary libraries
# include <string>
using namespace std;
int main()
{
int i = 1, u = 1, sum = 0, n; // Initialize variables i, u, sum, and n
cout << "\n\n Check whether a given number is a Perfect number:\n"; // Display message for user
cout << "------------------------------------------------------\n";
cout << "Input a number: "; // Ask user for input
cin >> n; // Get user input and store it in variable n
while (u <= n) // Loop through numbers from 1 to n
{
if (u < n) // Check if u is less than n
{
if (n % u == 0) // Check if u is a divisor of n
sum = sum + u; // Add u to the sum if it's a divisor of n
}
u++; // Increment u by 1 in each iteration
}
if (sum == n) // Check if the sum of divisors is equal to the number itself
{
cout << n << " is a Perfect number." << "\n"; // If the sum is equal to the number, it's a Perfect number
}
else
{
cout << n << " is not a Perfect number." << "\n"; // If the sum is not equal to the number, it's not a Perfect number
}
}
Sample Output:
Check whether a given number is a Perfect number: ------------------------------------------------------ Input a number: 28 28 is a Perfect number.
Flowchart:
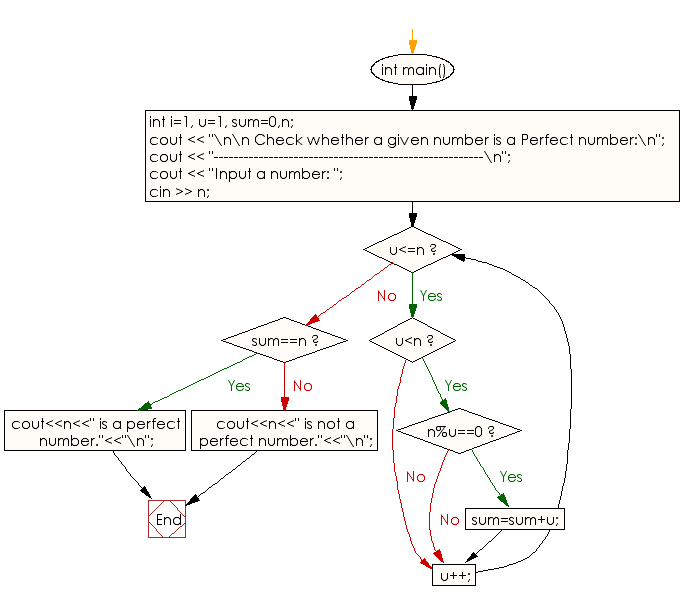
For more Practice: Solve these Related Problems:
- Write a C++ program to determine if a number is perfect by summing its proper divisors with an optimized loop.
- Write a C++ program to check if a number is perfect using recursion to compute the sum of its divisors.
- Write a C++ program that verifies perfect numbers by utilizing lambda expressions to encapsulate divisor summing.
- Write a C++ program to confirm if a number is perfect while also printing its divisors in sorted order.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to find the Abundant numbers (integers) between 1 to 1000.
Next: Write a program in C++ to find Perfect numbers and number of Perfect numbers between 1 to 1000.
What is the difficulty level of this exercise?