C++ Exercises: Print the first 20 numbers of the Pell series
39. Display First 20 Pell Numbers
Write a C++ program to print the first 20 numbers of the Pell series.
Sample Solution:
C++ Code :
#include <iostream> // Including the input-output stream library
using namespace std; // Using the standard namespace
int main() // Main function
{
int n, a = 1, b = 0, c; // Declare integer variables: n for iteration, a and b for Pell series calculation, c for storing the current value
cout << "\n\n Find the first 20 numbers of the Pell series: \n"; // Display purpose
cout << " --------------------------------------------------\n"; // Display purpose
cout << " The first 20 numbers of Pell series are: "<<endl; // Display purpose
c = 0; // Initializing the first value of Pell series
cout << " " << c << " "; // Displaying the first value of Pell series
for (n = 1; n < 20; n++) // Loop to generate the Pell series
{
c = a + 2 * b; // Calculate the Pell series value
cout << c << " "; // Display the current Pell series value
a = b; // Update 'a' with the previous value of 'b'
b = c; // Update 'b' with the current value of Pell series
}
cout << endl; // Output newline for better readability
}
Sample Output:
Find the first 20 numbers of the Pell series: -------------------------------------------------- The first 20 numbers of Pell series are: 0 1 2 5 12 29 70 169 408 985 2378 5741 13860 33461 80782 195025 470832 1136689 2744210 6625109
Flowchart:
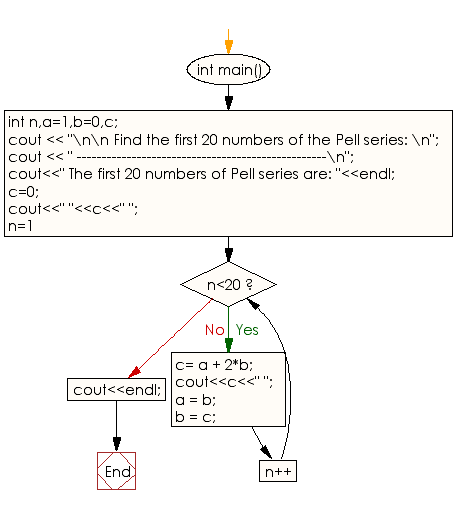
For more Practice: Solve these Related Problems:
- Write a C++ program to generate the first 20 Pell numbers using an iterative approach with dynamic programming.
- Write a C++ program to display Pell numbers by recursively computing each term with memoization.
- Write a C++ program to print Pell numbers with formatted output using STL containers for storage.
- Write a C++ program to compute and display the first 20 Pell numbers with careful handling of large integer values.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to check whether a given number is palindrome or not.
Next: Find Strong Numbers within a range of numbers.
What is the difficulty level of this exercise?