C++ Exercises: Find Narcissistic decimal numbers within a specific range
Write a C++ program to find Narcissistic decimal numbers within a specific range.
Sample Solution:
C++ Code :
#include <iostream> // Include input-output stream library
#include <cmath> // Include math library for mathematical operations
using namespace std; // Using standard namespace
int main() // Main function
{
int nl, nu; // Declare variables to store lower and upper limits
cout << "\n\n Find the Narcissistic decimal numbers between a specific range: \n"; // Display purpose
cout << " --------------------------------------------------------------------\n"; // Display purpose
cout << " Input the lower limit: "; // Prompt user for input
cin >> nl; // Input the lower limit
cout << " Input the upper limit: "; // Prompt user for input
cin >> nu; // Input the upper limit
cout << " The Narcissistic decimal numbers between " << nl << " and " << nu << " are: \n"; // Display purpose
int i, ctr, j, orn, n, m, sum; // Declare variables used in the following loops
for (orn = nl; orn <= nu; orn++) // Loop through the numbers within the range [nl, nu]
{
ctr = 0; // Initialize the counter to zero
sum = 0; // Initialize the sum to zero
n = orn; // Assign the current number 'orn' to 'n'
while (n > 0) // Loop to count the number of digits in 'n'
{
n = n / 10; // Divide 'n' by 10 to move to the next digit
ctr++; // Increment the digit counter
}
n = orn; // Assign the current number 'orn' to 'n' again for further processing
while (n > 0) // Loop to calculate the sum of digits raised to the power of the digit count
{
m = n % 10; // Extract the last digit of 'n'
sum = sum + pow(m, ctr); // Add the current digit raised to the power of the digit count to 'sum'
n = n / 10; // Move to the next digit by dividing 'n' by 10
}
if (sum == orn) // Check if the sum of digits raised to the power of the count is equal to the original number
{
cout << " " << orn << " "; // If equal, print the narcissistic number
}
}
cout << endl; // Move to the next line
}
Sample Output:
Find the Narcissistic decimal numbers between a specific range: -------------------------------------------------------------------- Input the lower limit: 25 Input a upper limit: 200 The Narcissistic decimal numbers between 25 and 200 are: 153
Flowchart:
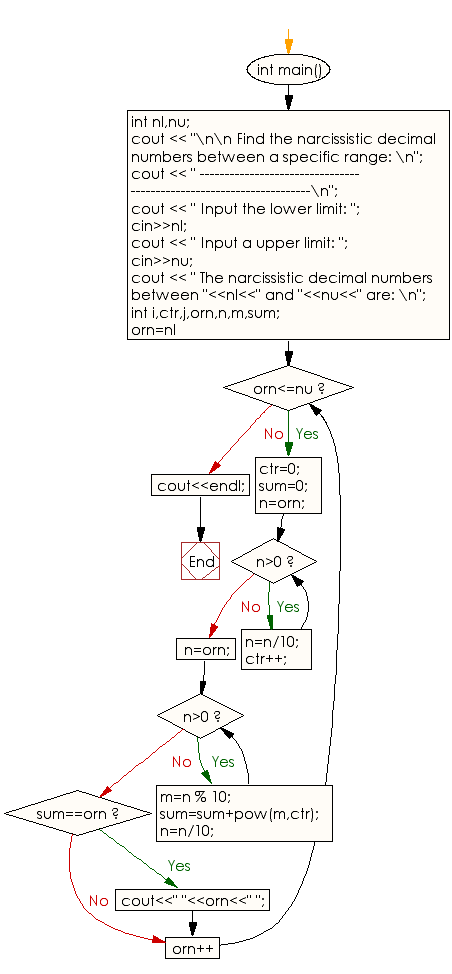
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to generate Mersenne primes within a range of numbers.
Next: Write a program in C++ to check whether a given number is palindrome or not.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics