C++ Exercises: Generate Mersenne primes within a range of numbers
36. Generate Mersenne Primes Within a Range
Write a C++ program to generate Mersenne primes within a range of numbers.
Sample Solution:
C++ Code :
#include<bits/stdc++.h> // Include entire standard library
using namespace std; // Using the standard namespace
void GenAllPrim(int n1, bool prarr1[]) // Function to generate all prime numbers until 'n1'
{
for (int i=0; i<=n1; i++) // Loop through array prarr1 and initialize all elements as true
prarr1[i] = true;
for (int p=2; p*p<=n1; p++) // Loop to mark non-prime numbers using Sieve of Eratosthenes algorithm
{
if (prarr1[p] == true) // If the number is marked as prime
{
for (int i=p*2; i<=n1; i += p) // Mark all multiples of 'p' as non-prime
prarr1[i] = false;
}
}
}
void chkMerPrime(int nm) // Function to check and print Mersenne prime numbers up to 'nm'
{
bool prarr1[nm+1]; // Declare an array to hold prime number information up to 'nm'
GenAllPrim(nm, prarr1); // Call function to generate prime numbers
for (int j=2; ((1<<j)-1) <= nm; j++) // Loop through potential Mersenne numbers: (2^j - 1)
{
long long num = (1<<j) - 1; // Calculate potential Mersenne number
if (prarr1[num]) // Check if the number is a prime number
cout <<" "<< num << " "; // If prime, print it as a Mersenne prime
}
}
int main() // Main function
{
int n; // Declare variable to store upper limit of the range
cout << "\n\n Generate Mersenne primes within a range of numbers:\n"; // Display purpose
cout << "--------------------------------------------------------\n"; // Display purpose
cout << " Input an upper limit [range from 1 to upper limit]: "; // Prompt user for input
cin >> n; // Input the upper limit
cout << " Mersenne prime numbers are: " << endl; // Display purpose
chkMerPrime(n); // Call function to check Mersenne primes within the given range
cout << endl << endl; // Display purpose
}
Sample Output:
Generate Mersenne primes within a range of numbers: -------------------------------------------------------- Input a upper limit [range from 1 to upper limit]: 200 Mersenne prime numbers are: 3 7 31 127
Flowchart:
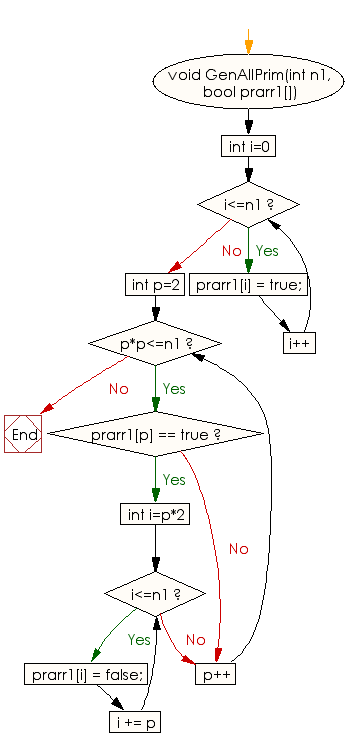
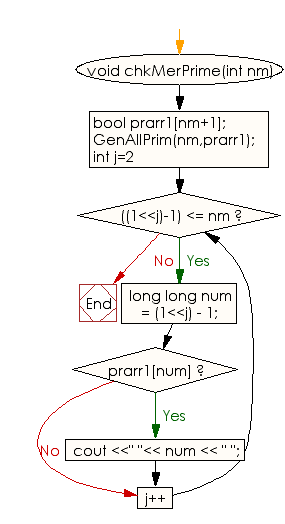
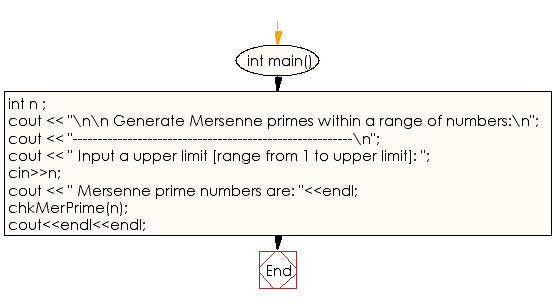
For more Practice: Solve these Related Problems:
- Write a C++ program to list all Mersenne primes below a user-specified upper limit using an efficient sieve.
- Write a C++ program to generate Mersenne primes within a range by combining prime testing with power-of-two checks.
- Write a C++ program to compute Mersenne primes using recursion and bitwise operators for performance.
- Write a C++ program to display Mersenne primes by iterating through exponents and testing for prime results.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to check if a number is Mersenne number or not.
Next: Write a program in C++ to find Narcissistic decimal numbers within a specific range.
What is the difficulty level of this exercise?