C++ Exercises: Find any number between 1 and n that can be expressed as the sum of two cubes in two different ways
34. Express Number as Sum of Two Cubes in Two Ways
Write a program in C++ to find any number between 1 and n that can be expressed as the sum of two cubes in two (or more) different ways.
Sample Solution:
C++ Code :
# include <iostream> // Including input-output stream library
# include <math.h> // Including math library for mathematical functions
using namespace std; // Using the standard namespace
int main() // Start of the main function
{
int a, b, c, d, n; // Declaring variables for looping and storage
double result; // Declaring a variable for storing the sum of cubes
cout << "\n\n Find the numbers between a range that can be expressed as the sum of two cubes:\n"; // Display purpose
cout << "------------------------------------------------------------------------------------\n"; // Display purpose
cout << " The numbers in the above range are: " << endl; // Display purpose
n = 100000; // Assigning the upper limit for the range
for (int a = 1; a <= n; a++) // Loop through potential values of 'a'
{
int a3 = a * a * a; // Calculate the cube of 'a'
if (a3 > n) break; // If the cube of 'a' exceeds the limit, exit loop
for (int b = a; b <= n; b++) // Loop through potential values of 'b'
{
int b3 = b * b * b; // Calculate the cube of 'b'
if (a3 + b3 > n) break; // If the sum of cubes of 'a' and 'b' exceeds the limit, exit loop
for (int c = a + 1; c <= n; c++) // Loop through potential values of 'c'
{
int c3 = c * c * c; // Calculate the cube of 'c'
if (c3 > a3 + b3) break; // If the cube of 'c' exceeds the sum of cubes of 'a' and 'b', exit loop
for (int d = c; d <= n; d++) // Loop through potential values of 'd'
{
int d3 = d * d * d; // Calculate the cube of 'd'
if (c3 + d3 > a3 + b3) break; // If the sum of cubes of 'c' and 'd' exceeds the sum of cubes of 'a' and 'b', exit loop
if (c3 + d3 == a3 + b3) // If the sum of cubes of 'c' and 'd' equals the sum of cubes of 'a' and 'b'
{
cout << " " << (a3 + b3) << " = "; // Output the sum of cubes
cout << a << "^3 + " << b << "^3 = "; // Output the equation for 'a' and 'b'
cout << c << "^3 + " << d << "^3"; // Output the equation for 'c' and 'd'
cout << endl; // Move to the next line for the next output
}
}
}
}
}
}
Sample Output:
Find the numbers between a range that can be expressed as the sum of two cubes: ------------------------------------------------------------------------------------ The numbers in the above range are: 1729 = 1^3 + 12^3 = 9^3 + 10^3 4104 = 2^3 + 16^3 = 9^3 + 15^3 13832 = 2^3 + 24^3 = 18^3 + 20^3 39312 = 2^3 + 34^3 = 15^3 + 33^3 46683 = 3^3 + 36^3 = 27^3 + 30^3 32832 = 4^3 + 32^3 = 18^3 + 30^3 40033 = 9^3 + 34^3 = 16^3 + 33^3 20683 = 10^3 + 27^3 = 19^3 + 24^3 65728 = 12^3 + 40^3 = 31^3 + 33^3 64232 = 17^3 + 39^3 = 26^3 + 36^3
Flowchart:
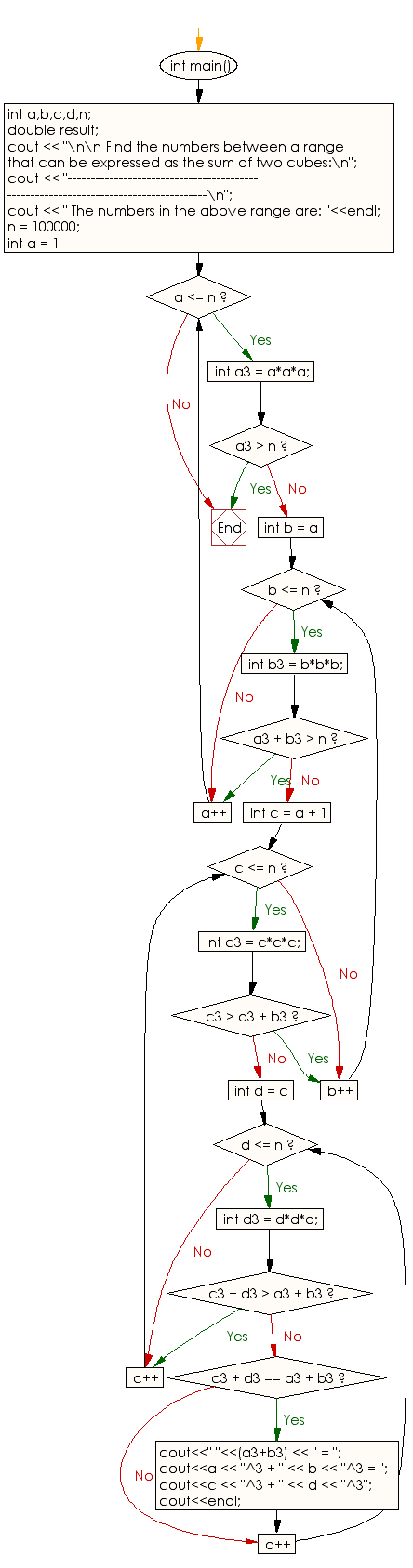
For more Practice: Solve these Related Problems:
- Write a C++ program to find numbers expressible as the sum of two cubes in two distinct ways using nested loops.
- Write a C++ program to list numbers with multiple representations as sums of cubes by precomputing cube values.
- Write a C++ program to generate and display numbers that can be written as the sum of two cubes in more than one way using STL containers.
- Write a C++ program to identify numbers with dual cube-sum representations by mapping sums to pairs of cubes.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to display first 10 Fermat numbers.
Next: Write a program in C++ to check if a number is Mersenne number or not.
What is the difficulty level of this exercise?