C++ Exercises: Check if a given number is circular prime or not
30. Circular Prime Check
Write a C++ program to check if a given number is circular prime or not.
Sample Solution:
C++ Code :
#include<iostream>
#include<cmath>
using namespace std;
int flg; // Global variable to track whether a number is prime or not
// Function to check if a number is prime
void chkPrime(long int n)
{
long int i = n - 1; // Initialize the variable i
// Loop to check for factors of the number 'n'
while (i >= 2)
{
if (n % i == 0) // If 'n' is divisible by 'i'
{
flg = 1; // Set flag to 1 indicating it's not a prime number
}
i--; // Decrement 'i'
}
}
// Function to generate all combinations of a number and check if they are prime
void AllCombination(long int a)
{
long int b1, c1, d1, e1, i, j, k, s1, z1, v1, x[8], y[8], m; // Declare variables
b1 = a; // Store the number 'a' in 'b1'
i = 0;
// Loop to break the number into its digits and store in an array
while (b1 > 0)
{
y[i] = b1 % 10; // Store the last digit of 'b1' in array 'y'
b1 = b1 / 10; // Remove the last digit
i++;
}
c1 = 0;
// Reversing the digits to create the combinations
for (j = i - 1; j >= 0; j--)
{
x[c1] = y[j]; // Store reversed digits in array 'x'
c1++;
}
m = i;
// Generating combinations and checking if they are prime
while (m > 0)
{
c1 = m - 1;
d1 = i - 1;
e1 = 0;
s1 = 0;
while (e1 < i)
{
z1 = pow(10, d1); // Calculate power of 10
v1 = z1 * x[c1 % i]; // Multiply power of 10 with digit
c1++;
d1--;
e1++;
s1 = s1 + v1; // Calculate the combined number
}
m--;
chkPrime(s1); // Check if the combined number is prime
}
}
int main()
{
long int num1;
cout << "\n\n Check whether a given number is circular prime or not: \n";
cout << " -----------------------------------------------------------\n";
cout << " Input a Number: ";
cin >> num1; // Input a number
flg = 0; // Initialize flag to 0
AllCombination(num1); // Call function to generate combinations
// Check if the number is circular prime or not based on the flag value
if (flg == 0)
{
cout << " The given number is a circular prime Number." << endl;
}
else
{
cout << " The given number is not a circular prime Number." << endl;
}
return 0; // Return from main function
}
Sample Output:
Check whether a given number is circular prime or not: ----------------------------------------------------------- Input a Number: 11 The given number is a circular prime Number
Flowchart:
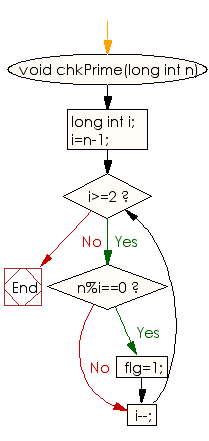
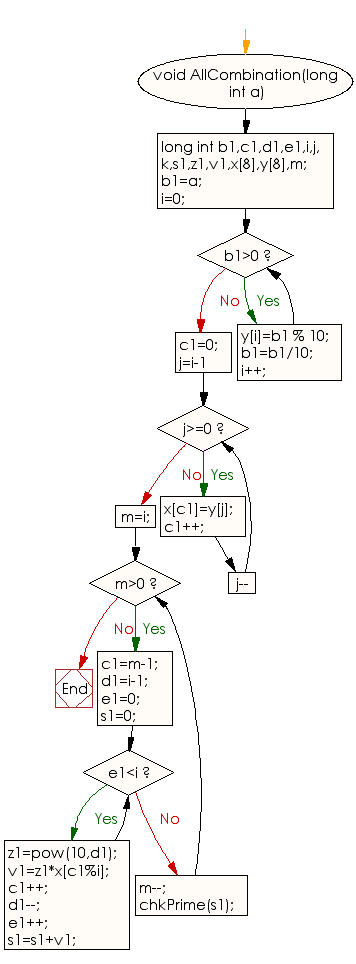
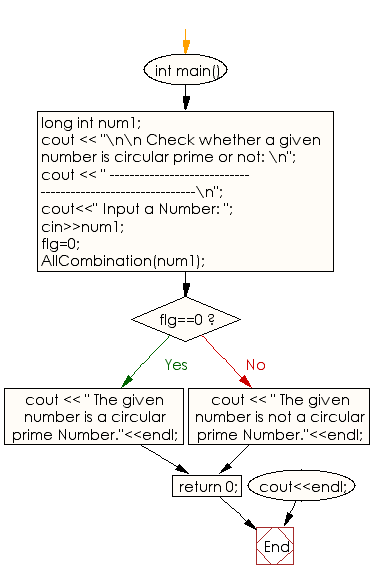
For more Practice: Solve these Related Problems:
- Write a C++ program to check if a number is a circular prime by rotating its digits and verifying primality.
- Write a C++ program to determine circular primes using string manipulation and a prime-checking function.
- Write a C++ program to verify circular prime status by implementing an efficient rotation algorithm.
- Write a C++ program to test for circular primes by converting numbers to strings and checking each rotation.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to count the Amicable pairs in an array.
Next: Write a program in C++ to find circular prime numbers upto a specific limit.
What is the difficulty level of this exercise?