C++ Exercises: Find the Abundant numbers between 1 to 1000
3. Find Abundant Numbers Between 1 and 1000
Write a program in C++ to find the Abundant numbers (integers) between 1 and 1000.
Visual Presentation:
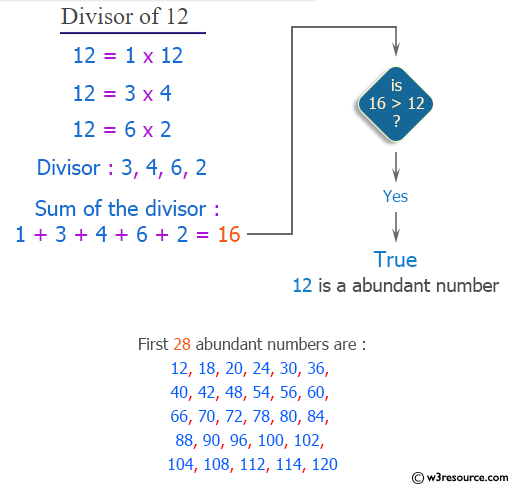
Sample Solution:
C++ Code :
#include <bits/stdc++.h> // Include necessary libraries
using namespace std;
// Function to get the sum of divisors of a number 'n'
int getSum(int n)
{
int sum = 0; // Initialize sum variable to store the sum of divisors
// Loop through numbers from 1 to square root of 'n'
for (int i = 1; i <= sqrt(n); i++)
{
if (n % i == 0) // Check if 'i' is a divisor of 'n'
{
if (n / i == i) // If divisor is same, add only 'i' to the sum
sum = sum + i;
else // Otherwise, add both divisors
{
sum = sum + i; // Add divisor 'i'
sum = sum + (n / i); // Add the other divisor ('n' divided by 'i')
}
}
}
sum = sum - n; // Subtract 'n' as it's not considered as a proper divisor
return sum; // Return the sum of divisors
}
// Function to check if a number is Abundant or not
bool checkAbundant(int n)
{
return (getSum(n) > n); // Return true if the sum of divisors is greater than 'n'
}
int main()
{
int n;
cout << "\n\n The Abundant number between 1 to 1000 are: \n"; // Display a message for the user
cout << " -----------------------------------------------\n";
// Loop through numbers from 1 to 1000 and check if each number is Abundant
for (int j = 1; j <= 1000; j++)
{
n = j; // Assign current number 'j' to variable 'n'
// If the number is Abundant, display it
checkAbundant(n) ? cout << n << " " : cout << "";
}
cout << endl; // Print newline after displaying Abundant numbers
return 0; // Return 0 to indicate successful completion of the program
}
Sample Output:
The Abundant number between 1 to 1000 are: ----------------------------------------------- 12 18 20 24 30 36 40 42 48 54 56 60 66 70 72 78 80 84 88 90 96 100 102 104 108 112 114 120 126 132 13 8 140 144 150 156 160 162 168 174 176 180 186 192 196 198 200 204 208 210 216 220 222 224 228 234 240 246 252 258 260 264 270 272 276 280 282 288 294 300 304 306 308 312 318 320 324 330 336 340 342 348 350 352 354 360 364 366 368 372 378 380 384 390 392 396 400 402 408 414 416 420 426 432 438 440 444 4 48 450 456 460 462 464 468 474 476 480 486 490 492 498 500 504 510 516 520 522 528 532 534 540 544 54 6 550 552 558 560 564 570 572 576 580 582 588 594 600 606 608 612 616 618 620 624 630 636 640 642 644 648 650 654 660 666 672 678 680 684 690 696 700 702 704 708 714 720 726 728 732 736 738 740 744 748 750 756 760 762 768 770 774 780 784 786 792 798 800 804 810 812 816 820 822 828 832 834 836 840 846 8 52 858 860 864 868 870 876 880 882 888 894 896 900 906 910 912 918 920 924 928 930 936 940 942 945 94 8 952 954 960 966 968 972 978 980 984 990 992 996 1000
Flowchart:
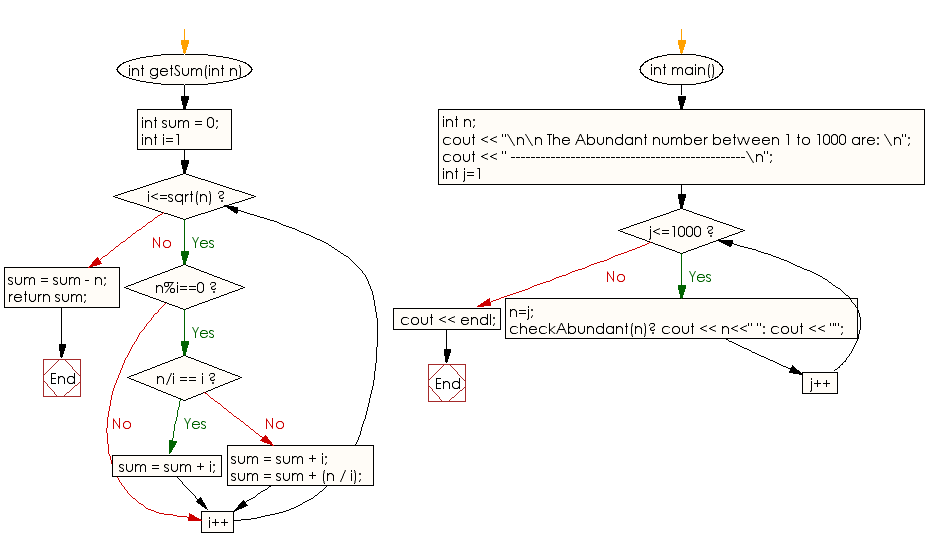
For more Practice: Solve these Related Problems:
- Write a C++ program to list all abundant numbers between 1 and 1000 using nested loops with early exit conditions.
- Write a C++ program to generate abundant numbers in a range by leveraging an efficient divisor-sum algorithm.
- Write a C++ program to compute and display abundant numbers between 1 and 1000 using vector storage and STL algorithms.
- Write a C++ program to find abundant numbers within a range by applying multi-threading to split the workload.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to check whether a given number is Abundant or not.
Next: Write a program in C++ to check whether a given number is Perfect or not.
What is the difficulty level of this exercise?