C++ Exercises: Count the Amicable pairs in an array
29. Count Amicable Pairs in an Array
Write a C++ program to count the amicable pairs in an array.
Sample Solution:
C++ Code :
#include <bits/stdc++.h> // Include all standard C++ libraries
using namespace std; // Using the standard namespace
int ProDivSum(int n)
{
int sum = 1; // Initializing sum with 1
for (int i = 2; i <= sqrt(n); i++) // Loop through numbers from 2 to square root of 'n'
{
if (n % i == 0) // Check if 'i' is a divisor of 'n'
{
sum += i; // Add 'i' to the sum
// To handle perfect squares, if 'n' is divisible by 'i' but not equal to 'i', add the corresponding divisor to the sum
if (n / i != i)
sum += n / i;
}
}
return sum; // Return the sum of proper divisors
}
bool chkAmicable(int a, int b)
{
return (ProDivSum(a) == b && ProDivSum(b) == a); // Return true if the sum of proper divisors of 'a' equals 'b' and vice versa
}
int ChkPairs(int arr[], int num1)
{
int ctr = 0; // Counter for amicable pairs
for (int i = 0; i < num1; i++) // Iterate through the array
{
for (int j = i + 1; j < num1; j++)
{
if (chkAmicable(arr[i], arr[j])) // Check if elements at index i and j form an amicable pair
ctr++; // Increment the counter if they are an amicable pair
}
}
return ctr; // Return the count of amicable pairs
}
int main()
{
int nn; // Declare variables
int n, i, j, ctr;
cout << "\n\n Count the Amicable pairs in a specific array: \n"; // Display a message
cout << " Sample pairs : (220, 284)(1184,1210) (2620,2924) (5020,5564) (6232,6368)... \n"; // Display sample pairs
cout << " ------------------------------------------------------------------------------\n";
cout << "\n Input the number of elements to be stored in the array: ";
cin >> nn; // Input the number of elements in the array
int arr1[nn]; // Declare an array of size nn
for (i = 0; i < nn; i++) // Input the elements of the array
{
cout << "element - " << i << ": ";
cin >> arr1[i];
}
int n1 = sizeof(arr1) / sizeof(arr1[0]); // Calculate the number of elements in the array
cout << " Number of Amicable pairs present in the array: " << ChkPairs(arr1, n1) << endl; // Count and display the number of amicable pairs in the array
return 0; // Exit the program
}
Sample Output:
Count the Amicable pairs in a specific array: Sample pairs : (220, 284)(1184,1210) (2620,2924) (5020,5564) (6232,6368)... ------------------------------------------------------------------------------ Input the number of elements to be stored in the array: 2 element - 0: 220 element - 1: 284 Number of Amicable pairs presents in the array: 1
Flowchart:
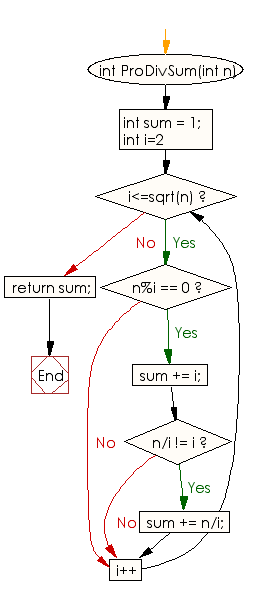
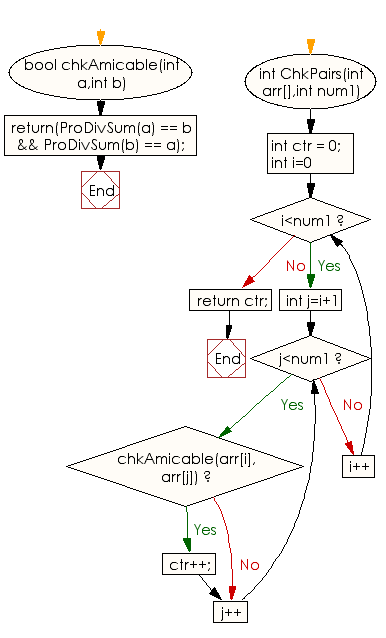
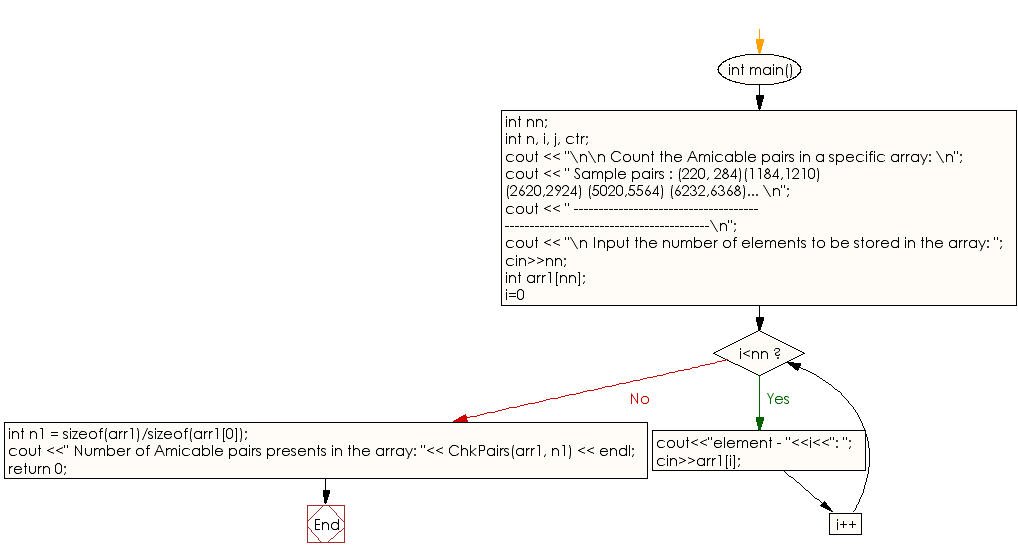
For more Practice: Solve these Related Problems:
- Write a C++ program to count amicable pairs within an array by checking every possible pair with nested loops.
- Write a C++ program to identify and count amicable pairs in an array using STL algorithms and custom predicates.
- Write a C++ program to compute amicable pairs in an array by mapping each number to its divisor sum and comparing.
- Write a C++ program to count amicable pairs from an array with error checking for duplicate pairings.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to check two numbers are Amicable numbers or not.
Next: Write a program in C++ to check if a given number is circular prime or not.
What is the difficulty level of this exercise?