C++ Exercises: Find Duck Numbers between 1 to 500
Write a C++ program to find Duck Numbers between 1 and 500.
Visual Presentation:
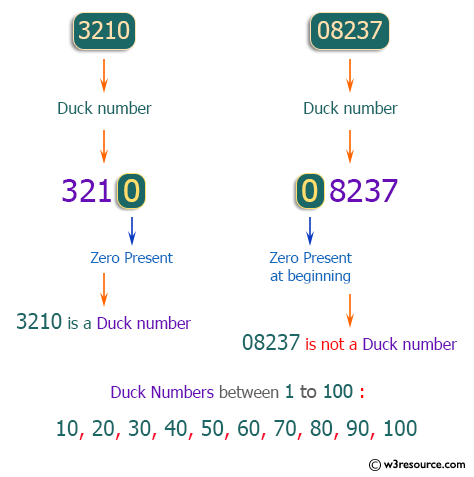
Sample Solution:
C++ Code :
#include<bits/stdc++.h> // Include all standard C++ libraries
using namespace std; // Using the standard namespace
int main()
{
int dno, dkno, r, flg; // Declare integer variables
flg = 0; // Initialize flag variable
cout << "\n\n Find Duck Numbers between 1 to 500: \n"; // Display a message
cout << " ----------------------------------------\n";
cout << " The Duck numbers are: " << endl;
for (dkno = 1; dkno <= 500; dkno++) // Loop through numbers from 1 to 500
{
dno = dkno; // Store the current number in 'dno'
flg = 0; // Reset the flag for each iteration
while (dno > 0) // Loop to check if there is any zero digit in the number
{
if (dno % 10 == 0) // If the last digit is zero
{
flg = 1; // Set flag to indicate the presence of zero
break; // Exit the loop
}
dno /= 10; // Move to the next digit by removing the last digit
}
if (dkno > 0 && flg == 1) // Check if the number is positive and contains a zero
{
cout << dkno << " "; // Display the Duck Number
}
}
cout << endl;
}
Sample Output:
Find Duck Numbers between 1 to 500: ---------------------------------------- The Duck numbers are: 10 20 30 40 50 60 70 80 90 100 101 102 103 104 105 106 107 108 109 110 120 130 140 150 160 170 180 190 200 201 202 203 204 205 206 207 208 209 210 220 230 240 250 260 270 280 290 300 301 302 303 304 305 306 307 30 8 309 310 320 330 340 350 360 370 380 390 400 401 402 403 404 405 406 4 07 408 409 410 420 430 440 450 460 470 480 490 500
Flowchart:
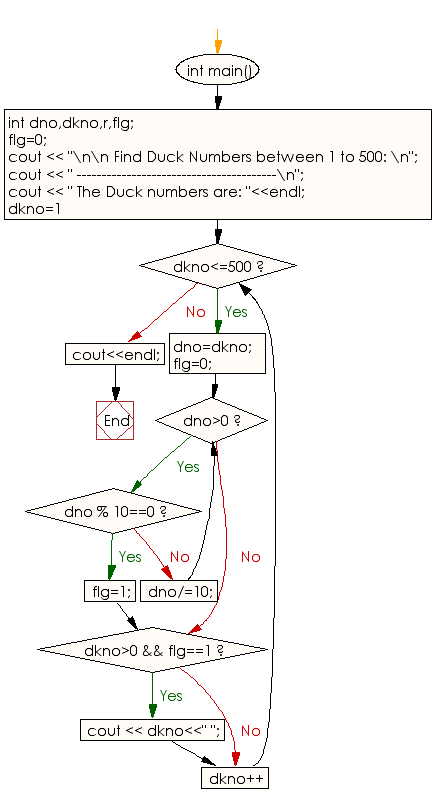
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to check whether a number is a Duck Number or not.
Next: Write a program in C++ to check two numbers are Amicable numbers or not.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics