C++ Exercises: Check whether a number is a Duck Number or not
26. Duck Number Check
Write a C++ program to check whether a number is a Duck Number or not.
Visual Presentation:
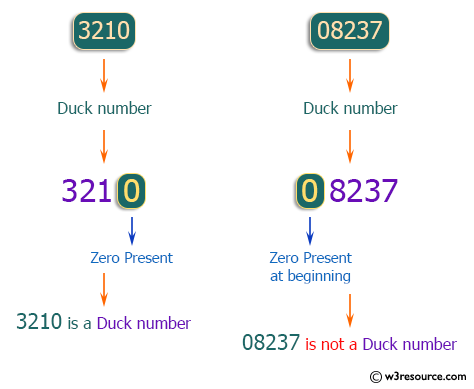
Sample Solution:
C++ Code :
#include <bits/stdc++.h> // Includes all standard C++ libraries
using namespace std; // Using the standard namespace
int main()
{
int dno, dkno, r, flg; // Declaring integer variables
flg = 0; // Initializing flag variable
cout << "\n\n Check whether a number is a Duck Number or not: \n"; // Displaying a message
cout << " ----------------------------------------------------\n";
cout << " Input a number: ";
cin >> dkno; // Taking input from the user
dno = dkno; // Storing the original number in 'dno'
while (dkno > 0) // Loop to check if there is any zero digit in the number
{
if (dkno % 10 == 0) // If the last digit is zero
{
flg = 1; // Set flag to indicate the presence of zero
break; // Exit the loop
}
dkno /= 10; // Move to the next digit by removing the last digit
}
if (dno > 0 && flg == 1) // Checking if the original number is positive and if there is a zero digit
{
cout << " The given number is a Duck Number." << endl; // Display if it's a Duck Number
}
else
{
cout << " The given number is not a Duck Number." << endl; // Display if it's not a Duck Number
}
}
Sample Output:
Check whether a number is a Duck Number or not: ---------------------------------------------------- Input a number: 30 The given number is a Duck Number.
Flowchart:
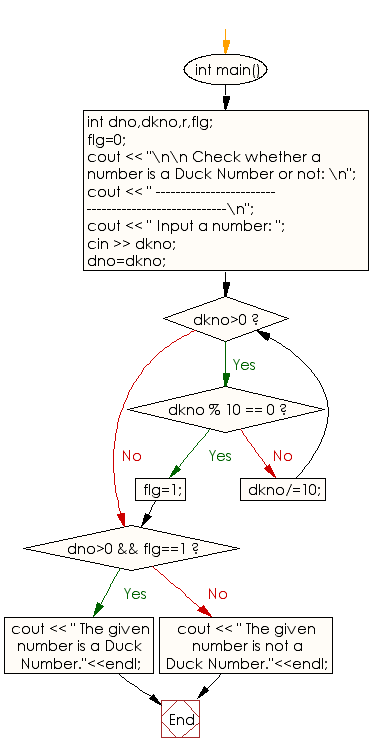
For more Practice: Solve these Related Problems:
- Write a C++ program to check if a number is a duck number by ensuring it contains zero but not as the first digit.
- Write a C++ program to verify duck numbers using string scanning and pattern matching techniques.
- Write a C++ program to determine duck numbers with careful consideration for edge cases like leading zeros.
- Write a C++ program to test for duck numbers by converting the number to a character array and scanning for '0'.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to find the Authomorphic numbers between 1 to 1000.
Next: Write a program in C++ to find Duck Numbers between 1 to 500.
What is the difficulty level of this exercise?