C++ Exercises: Find the Authomorphic numbers between 1 to 1000
25. Find Automorphic Numbers Between 1 and 1000
Write a C++ program to find the Authomorphic numbers between 1 and 1000.
Sample Solution:
C++ Code :
#include<iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function to check if a number is an Automorphic Number
bool chkAutomor(int num1)
{
int sqno = num1 * num1; // Computing the square of the input number
while (num1 > 0) // Loop to check the digits
{
if (num1 % 10 != sqno % 10) // Checking the last digits
return false; // If not equal, it's not an Automorphic Number
num1 /= 10; // Move to the next digit in the input number
sqno /= 10; // Move to the next digit in the square of the input number
}
return true; // If all digits match, it's an Automorphic Number
}
// Main function
int main()
{
int i; // Variable for iteration
cout << "\n\n Find the the Authomorphic numbers between 1 to 1000 \n"; // Displaying a message
cout << " -------------------------------------------------------\n";
cout << " The Authomorphic numbers are: "<<endl;
// Checking numbers from 1 to 1000 for being Automorphic Numbers and displaying the result
for(i=1;i<=1000;i++)
{
if( chkAutomor(i))
cout << i<<" "; // Displaying the number if it's an Automorphic Number
}
cout<<endl;
return 0; // Return 0 to indicate successful completion
}
Sample Output:
Find the the Authomorphic numbers between 1 to 1000 ------------------------------------------------------- The Authomorphic numbers are: 1 5 6 25 76 376 625
Flowchart:
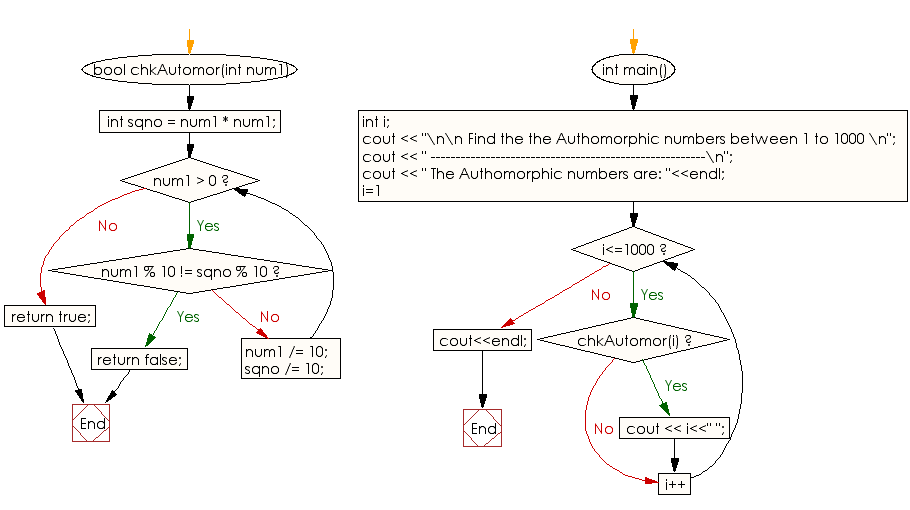
For more Practice: Solve these Related Problems:
- Write a C++ program to list all automorphic numbers in a given range using iterative comparison of string endings.
- Write a C++ program to generate automorphic numbers between 1 and 1000 using mathematical modulus operations.
- Write a C++ program to display automorphic numbers by precomputing squares and comparing digit sequences.
- Write a C++ program to identify automorphic numbers with robust input validation and formatted output.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to check if a number is Authomorphic or not.
Next: Write a program in C++ to check whether a number is a Duck Number or not.
What is the difficulty level of this exercise?