C++ Exercises: Check if a number is Authomorphic or not
Write a program in C++ to check if a number is Authomorphic or not.
Sample Solution:
C++ Code :
#include<iostream> // Including the input/output stream library
using namespace std; // Using the standard namespace
// Function to check if a number is an Automorphic Number
bool chkAutomor(int num1)
{
int sqno = num1 * num1; // Computing the square of the input number
while (num1 > 0) // Loop to check the digits
{
if (num1 % 10 != sqno % 10) // Checking the last digits
return false; // If not equal, it's not an Automorphic Number
num1 /= 10; // Move to the next digit in the input number
sqno /= 10; // Move to the next digit in the square of the input number
}
return true; // If all digits match, it's an Automorphic Number
}
// Main function
int main()
{
int auno; // Declaring variable to store user input
cout << "\n\n Check whether a number is an Automorphic Number or not: \n"; // Displaying a message
cout << " ------------------------------------------------------------\n";
cout << " Input a number: ";
cin >> auno; // Taking user input
// Checking if the entered number is an Automorphic Number and displaying the result
if( chkAutomor(auno))
cout << " The given number is an Automorphic Number."<<endl;
else
cout << " The given number is not an Automorphic Number."<<endl;
return 0; // Return 0 to indicate successful completion
}
Sample Output:
Check whether a number is an Authomorphic Number or not: ------------------------------------------------------------ Input a number: 25 The given number is an Automorphic Number.
Flowchart:
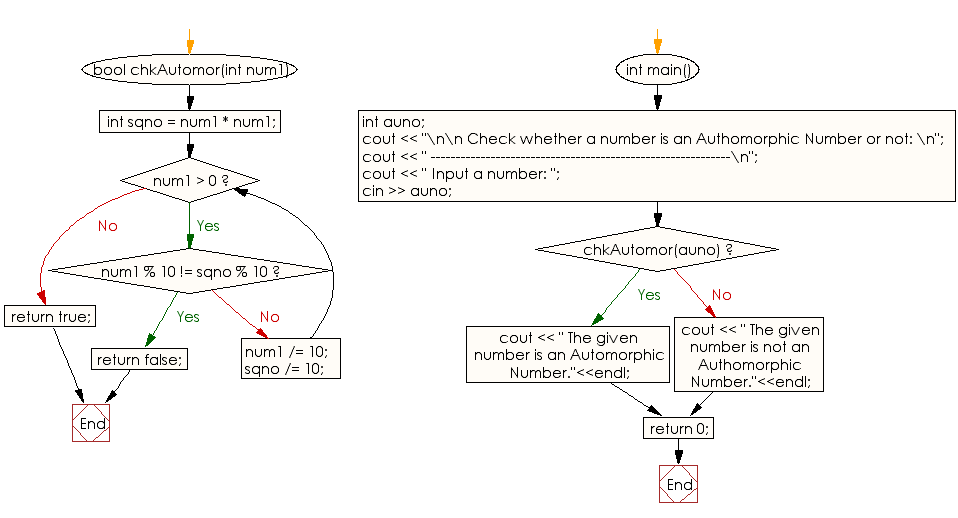
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to find Pronic Number between 1 to 1000.
Next: Write a program in C++ to find the Authomorphic numbers between 1 to 1000.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics