C++ Exercises: Find Pronic Number between 1 to 1000
23. Find Pronic Numbers Between 1 and 1000
Write a program in C++ to find Pronic numbers between 1 and 1000.
Sample Solution:
C++ Code :
#include<bits/stdc++.h> // Include necessary libraries
using namespace std; // Use the standard namespace
int main()
{
int prno, i, n, flg; // Declare variables
cout << "\n\n Find the Pronic Numbers between 1 to 1000: \n"; // Display a message
cout << " -----------------------------------------------\n";
cout << " The Pronic numbers are: " << endl; // Display a message
// Loop through numbers from 1 to 1000 to find Pronic Numbers
for (prno = 1; prno <= 1000; prno++)
{
flg = 0; // Initialize flag to 0
// Loop to check if the current number is a Pronic Number
for (i = 1; i <= prno; i++)
{
if (i * (i + 1) == prno) // Check if the number is a product of consecutive integers
{
flg = 1; // Set flag to 1 if it is a Pronic Number
break; // Exit the loop if a Pronic Number is found
}
}
// Check if the flag is set, indicating that the number is a Pronic Number
if (flg == 1)
{
cout << prno << " "; // Display the Pronic Number
}
}
cout << endl; // Move to the next line after printing all Pronic Numbers
}
Sample Output:
Find the Pronic Numbers between 1 to 1000: ----------------------------------------------- The Pronic numbers are: 2 6 12 20 30 42 56 72 90 110 132 156 182 210 240 272 306 342 380 420 462 506 552 600 650 702 756 812 870 930 992
Flowchart:
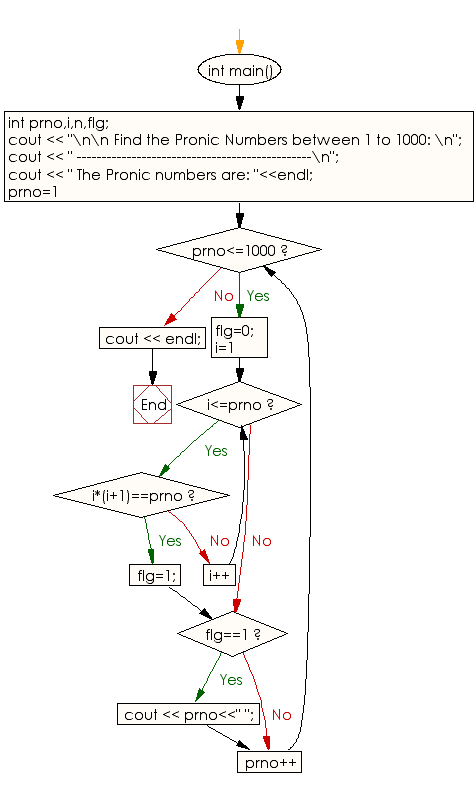
For more Practice: Solve these Related Problems:
- Write a C++ program to list all pronic numbers in a range by computing products of consecutive integers.
- Write a C++ program to generate pronic numbers between 1 and 1000 using a for-loop with optimized calculations.
- Write a C++ program to display pronic numbers by precomputing and storing results in a vector.
- Write a C++ program to find pronic numbers within a range with error-checking for input validity.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to check whether a number is a Pronic Number or Heteromecic Number or not.
Next: Write a program in C++ to check if a number is Authomorphic or not.
What is the difficulty level of this exercise?