C++ Exercises: Find Harshad Number between 1 to 100
C++ Numbers: Exercise-21 with Solution
Write a C++ program to find the Harshad numbers between 1 and 100.
Sample Solution:
C++ Code :
#include<bits/stdc++.h> // Include necessary libraries
using namespace std; // Use the standard namespace
// Function to check if a number is a Harshad Number
bool chkHarshad(int n)
{
int s = 0; // Initialize a variable to store the sum of digits
int tmp; // Temporary variable to hold the number during the loop iterations
// Loop to calculate the sum of digits of the number
for (tmp = n; tmp > 0; tmp /= 10)
s += tmp % 10; // Extract the last digit of the number and add it to the sum
return (n % s == 0); // Return true if the number is divisible by the sum of its digits (Harshad Number condition)
}
// Main function
int main()
{
int i; // Variable for loop iteration
cout << "\n\n Find Harshad Numbers between 1 to 100: \n"; // Display a message about the program
cout << " ---------------------------------------------------\n";
cout << " The Harshad Numbers are: " << endl; // Display a message
// Loop through numbers from 1 to 100 to find Harshad Numbers
for (i = 1; i <= 100; i++)
{
if (chkHarshad(i)) // Check if the number is a Harshad Number using the chkHarshad function
cout << i << " "; // Display the number if it is a Harshad Number
}
cout << endl; // Move to the next line
return 0; // Return 0 to indicate successful execution
}
Sample Output:
Find Harshad Numbers between 1 to 100: --------------------------------------------------- The Harshad Numbers are: 1 2 3 4 5 6 7 8 9 10 12 18 20 21 24 27 30 36 40 42 45 48 50 54 60 63 70 72 80 81 84 90 100
Flowchart:
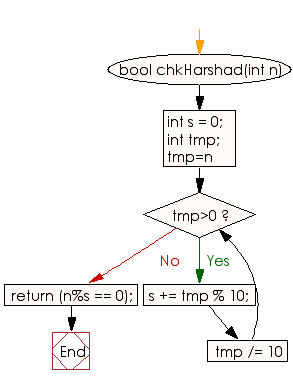
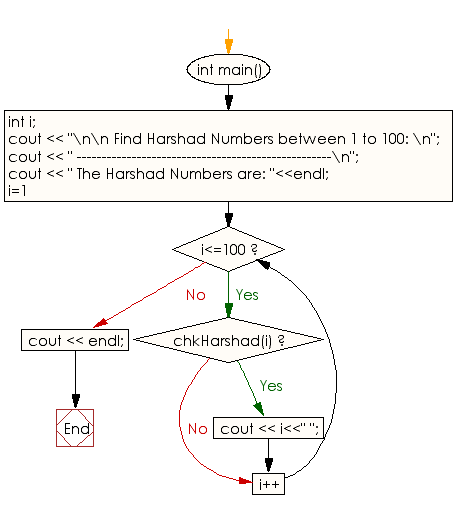
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to check if a number is Harshad Number or not.
Next: Write a program in C++ to check whether a number is a Pronic Number or Heteromecic Number or not.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics