C++ Exercises: Check if a number is Harshad Number or not
C++ Numbers: Exercise-20 with Solution
Write a C++ program to check if a number is a Harshad Number or not.
Sample Solution:
C++ Code :
#include<bits/stdc++.h> // Include necessary libraries
using namespace std; // Use the standard namespace
// Function to check if a number is a Harshad Number
bool chkHarshad(int n)
{
int s = 0; // Initialize a variable to store the sum of digits
int tmp; // Temporary variable to hold the number during the loop iterations
// Loop to calculate the sum of digits of the number
for (tmp = n; tmp > 0; tmp /= 10)
s += tmp % 10; // Extract the last digit of the number and add it to the sum
return (n % s == 0); // Return true if the number is divisible by the sum of its digits (Harshad Number condition)
}
// Main function
int main()
{
int hdno; // Variable to store the user input
cout << "\n\n Check whether a number is Harshad Number or not: \n"; // Display a message about the program
cout << " ---------------------------------------------------\n";
cout << " Input a number: "; // Prompt the user to input a number
cin >> hdno; // Read the user input
if (chkHarshad(hdno)) // Check if the number is a Harshad Number using the chkHarshad function
cout << " The given number is a Harshad Number." << endl; // Display message if the number is a Harshad Number
else
cout << " The given number is not a Harshad Number." << endl; // Display message if the number is not a Harshad Number
return 0; // Return 0 to indicate successful execution
}
Sample Output:
Check whether a number is Harshad Number or not: --------------------------------------------------- Input a number: 18 The given number is a Harshad Number.
Flowchart:
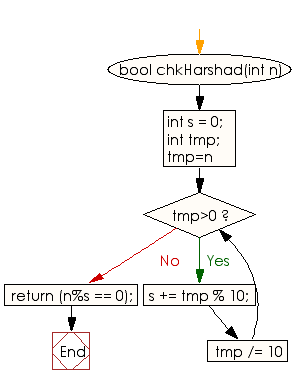
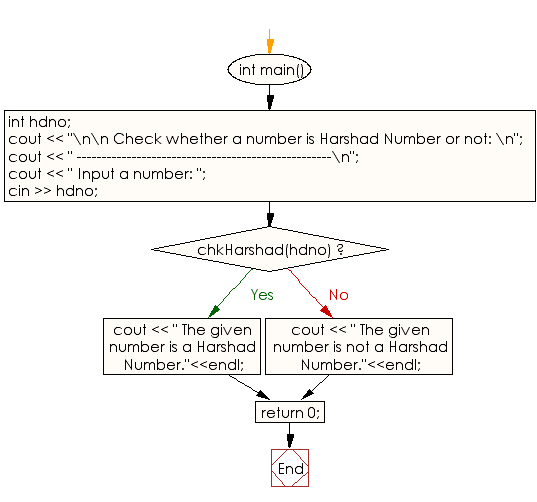
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to find Disarium numbers between 1 to 1000.
Next: Write a program in C++ to find Harshad Number between 1 to 100.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/cpp-exercises/numbers/cpp-numbers-exercise-20.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics