C++ Exercises: Check whether a number is Disarium or not
18. Disarium Number Check
Write a C++ program to check whether a number is Disarium or not.
Visual Presentation:
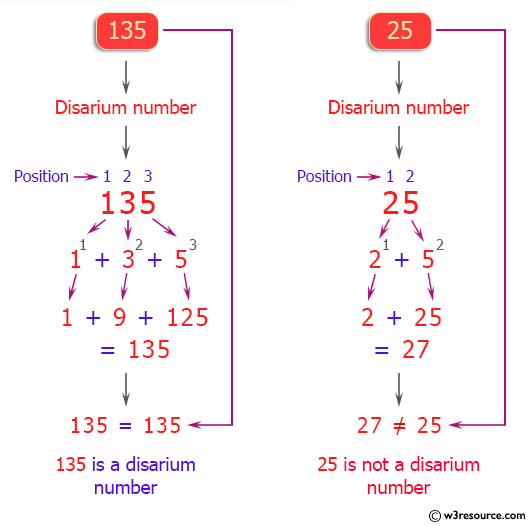
Sample Solution:
C++ Code :
#include<bits/stdc++.h> // Include necessary libraries
using namespace std; // Use the standard namespace
// Function to count the number of digits in a number
int DigiCount(int n)
{
int ctr_digi = 0; // Counter for digits initialized to 0
int tmpx = n; // Temporary variable to store the number
// Loop to count the digits by dividing the number by 10 until it becomes zero
while (tmpx)
{
tmpx = tmpx / 10; // Divide the number by 10
ctr_digi++; // Increment digit counter
}
return ctr_digi; // Return the count of digits in the number
}
// Function to check if a number is a Disarium Number
bool chkDisarum(int n)
{
int ctr_digi = DigiCount(n); // Calculate the count of digits in the number
int s = 0; // Initialize sum of powered digits to 0
int x = n; // Temporary variable to store the number
int pr; // Variable to store digits extracted from the number
// Loop to calculate the sum of powered digits
while (x)
{
pr = x % 10; // Extract the last digit of the number
s = s + pow(pr, ctr_digi--); // Add the powered digit to the sum
x = x / 10; // Remove the last digit from the number
}
return (s == n); // Return true if the sum of powered digits is equal to the original number
}
// Main function
int main()
{
int dino; // Variable to store user input
cout << "\n\n Check whether a number is Disarium Number or not: \n"; // Display message about the program
cout << " ---------------------------------------------------\n";
cout << " Input a number: "; // Ask user to input a number
cin >> dino; // Take user input
// Check if the input number is a Disarium Number and display the result
if (chkDisarum(dino))
cout << " The given number is a Disarium Number." << endl;
else
cout << " The given number is not a Disarium Number." << endl;
return 0; // Return 0 to indicate successful execution
}
Sample Output:
Check whether a number is Disarium Number or not: --------------------------------------------------- Input a number: 9 The given number is a Disarium Number.
Flowchart:
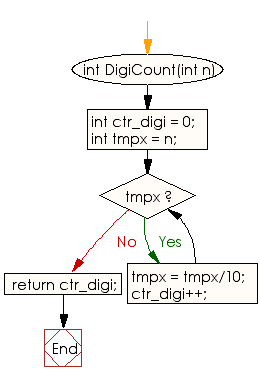
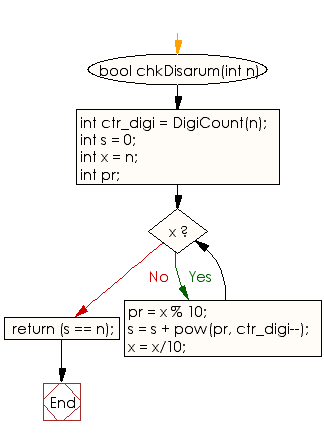
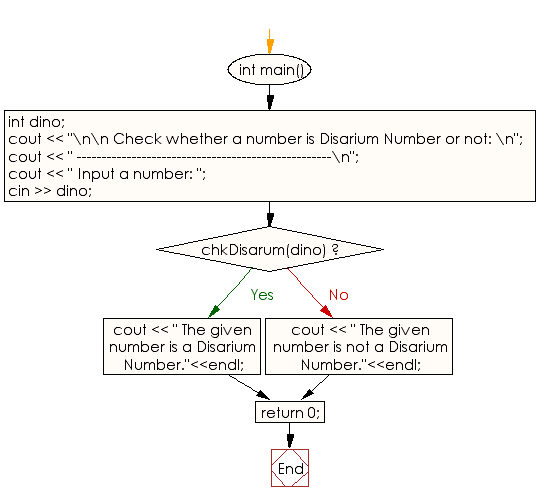
For more Practice: Solve these Related Problems:
- Write a C++ program to check if a number is Disarium by summing its digits raised to their respective positions.
- Write a C++ program to determine Disarium numbers using string conversion for digit extraction.
- Write a C++ program to verify a Disarium number by processing its digits with a for-loop and power function.
- Write a C++ program to test Disarium numbers with error handling for non-positive integers.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to find the Happy numbers between 1 to 1000.
Next: Write a program in C++ to find Disarium numbers between 1 to 1000.
What is the difficulty level of this exercise?