C++ Exercises: Display the first 10 Catlan numbers
15. Display First 10 Catalan Numbers
Write a program in C++ to display the first 10 Catlan numbers.
Visual Presentation:
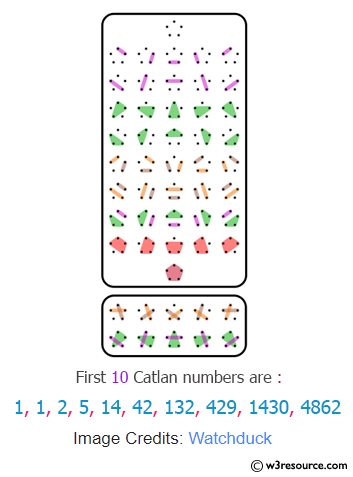
Sample Solution:
C++ Code :
#include<iostream> // Include the input/output stream library
using namespace std; // Use the standard namespace
// Function to calculate the Catalan number for a given 'n'
unsigned long int cataLan(unsigned int n)
{
// Base case: If 'n' is 0 or 1, return 1 (Catalan number definition)
if (n <= 1)
return 1;
unsigned long int catno = 0; // Initialize Catalan number variable
// Loop to calculate Catalan number using the recursive formula
for (int i = 0; i < n; i++)
catno += cataLan(i) * cataLan(n - i - 1); // Recursive calculation for Catalan number
return catno; // Return the calculated Catalan number for 'n'
}
// Main function
int main()
{
cout << "\n\n Find the first 10 Catalan numbers: \n"; // Display message for the purpose of the program
cout << " --------------------------------------\n";
cout << " The first 10 Catalan numbers are: "<<endl; // Display message for the result
// Loop to print the first 10 Catalan numbers
for (int i = 0; i < 10; i++)
cout << cataLan(i) << " "; // Display each Catalan number in the sequence
cout << endl; // Move to a new line for better readability
return 0; // Return 0 to signify successful completion of the program
}
Sample Output:
Find the first 10 Catlan numbers: -------------------------------------- The first 10 Catlan numbers are: 1 1 2 5 14 42 132 429 1430 4862
Flowchart:
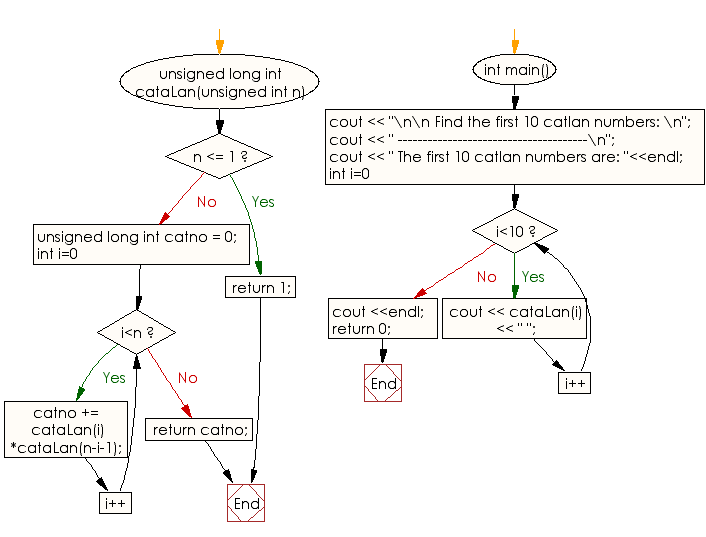
For more Practice: Solve these Related Problems:
- Write a C++ program to compute the first 10 Catalan numbers using recursion and dynamic programming.
- Write a C++ program to generate Catalan numbers with memoization and display them in formatted output.
- Write a C++ program to calculate Catalan numbers iteratively with efficient use of arrays for storage.
- Write a C++ program to display the first 10 Catalan numbers using a combinatorial formula and precomputed factorials.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to display the first 10 Lucus numbers.
Next: Write a program in C++ to check a number is a Happy or not.
What is the difficulty level of this exercise?