C++ Exercises: Display the first 10 Lucus numbers
14. Display First 10 Lucas Numbers
Write a program in C++ to display the first 10 Lucus numbers.
Visual Presentation:
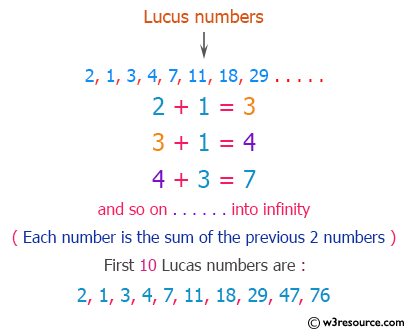
Sample Solution:
C++ Code :
#include <iostream> // Include the input/output stream library
#include <cmath> // Include the math library for mathematical operations
using namespace std; // Use the standard namespace
int main()
{
// Display a message indicating to find the first 10 Lucas numbers
cout << "\n\n Find the first 10 Lucas numbers: \n";
cout << " -------------------------------------\n";
// Display a message indicating the result will be the first 10 Lucas numbers
cout << " The first 10 Lucas numbers are: "<<endl;
int n = 10; // Define the count of Lucas numbers to find
int n1 = 2, n2 = 1, n3; // Initialize the first two Lucas numbers
// Check if the input count of numbers is greater than 1
if (n > 1)
{
// Display the first two Lucas numbers
cout << n1 << " " << n2 << " ";
// Loop to generate and display the subsequent Lucas numbers
for (int i = 2; i < n; ++i)
{
n3 = n2; // Store the current value of n2 in n3
n2 += n1; // Calculate the next Lucas number by adding n1 to n2
n1 = n3; // Set the new value of n1 as the previous value of n2
cout << n2 << " "; // Display the next Lucas number
}
cout << endl; // Move to a new line for better readability
}
else if (n == 1) // Check if the input count is 1
{
cout << n2 << " "; // If so, display the first Lucas number
cout << endl; // Move to a new line for better readability
}
else
{
// If the input is less than 1, display a message to input a positive number
cout << "Input a positive number." << endl;
}
}
Sample Output:
Find the first 10 Lucus numbers: ------------------------------------- The first 10 Lucus numbers are: 2 1 3 4 7 11 18 29 47 76
Flowchart:
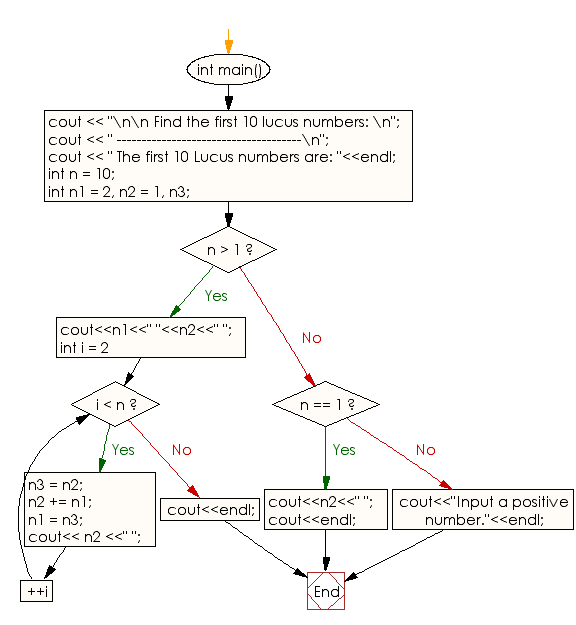
For more Practice: Solve these Related Problems:
- Write a C++ program to generate the first 10 Lucas numbers using an iterative loop with memoization.
- Write a C++ program to compute Lucas numbers recursively with tail recursion optimization.
- Write a C++ program to display Lucas numbers using an array to store computed values for fast lookup.
- Write a C++ program to generate the Lucas series with error handling for potential integer overflow.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to generate and show the first 15 Narcissistic decimal numbers.
Next: Write a program in C++ to display the first 10 Catlan numbers.
What is the difficulty level of this exercise?