C++ Exercises: Generate and show the first 15 Narcissistic decimal numbers
Write a program in C++ to generate and show the first 15 Narcissistic decimal numbers.
Visual Presentation:
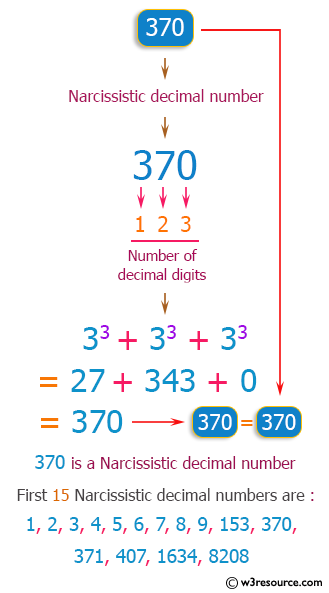
Sample Solution:
C++ Code :
#include <iostream> // Include the input/output stream library
#include <cmath> // Include the math library for mathematical operations
using namespace std; // Use the standard namespace
int main()
{
// Display a message for the user to find the first 15 Narcissistic decimal numbers
cout << "\n\n Find the first 15 Narcissistic decimal numbers: \n";
cout << " -----------------------------------------------------\n";
// Display a message indicating the result will be the first 15 Narcissistic decimal numbers
cout << " The first 15 Narcissistic decimal numbers are: \n";
int i, ctr, j, orn, n, m, sum; // Declare integer variables for iteration and calculations
orn = 1; // Set the initial number to check as 1
// Loop to find the first 15 Narcissistic decimal numbers
for (i = 1; i <= 15;)
{
ctr = 0; // Initialize a counter for the number of digits in orn
sum = 0; // Initialize a variable to store the sum of powers of digits
n = orn; // Store the current number to check Narcissistic property
// Calculate the number of digits (order) in the number
while (n > 0)
{
n = n / 10; // Reduce the number by one digit
ctr++; // Increment the counter to count the digits
}
n = orn; // Reset the number for calculations
// Calculate the sum of the powers of digits raised to the order
while (n > 0)
{
m = n % 10; // Extract the last digit of the number
sum = sum + pow(m, ctr); // Add the m^ctr to the sum
n = n / 10; // Reduce the number by one digit
}
// Check if the sum of powers of digits equals the original number (orn)
if (sum == orn)
{
cout << orn << " "; // If true, display the number as Narcissistic
i++; // Increment the counter for found Narcissistic numbers
}
orn++; // Increment the original number for the next iteration
}
cout << endl; // Add a newline for better readability
}
Sample Output:
Find the first 15 narcissistic decimal numbers: ----------------------------------------------------- The first 15 narcissistic decimal numbers are: 1 2 3 4 5 6 7 8 9 153 370 371 407 1634 8208
Flowchart:
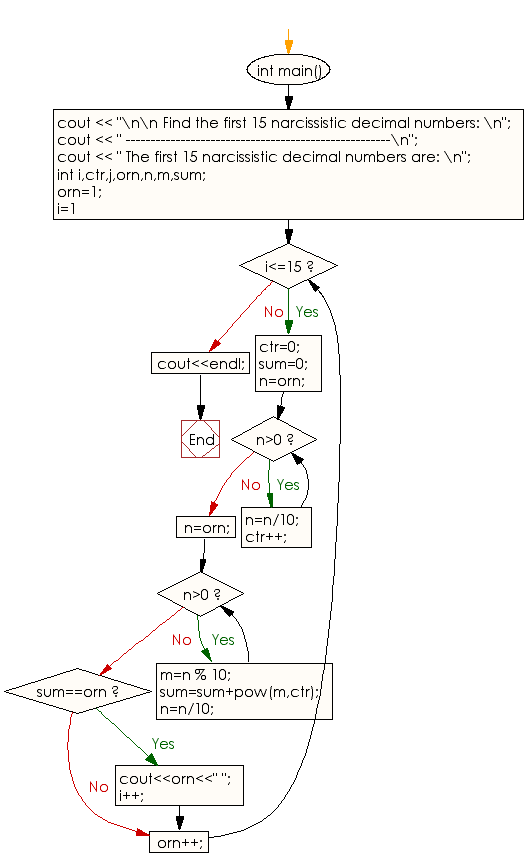
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to find the Lychrel numbers and the number of Lychrel number within the range 1 to 1000 (after 500 iteration).
Next: Write a program in C++ to display the first 10 Lucus numbers.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics