C++ Exercises: Check whether a given number is an Ugly number or not
1. Ugly Number Check
Write a program in C++ to check whether a given number is an Ugly number or not.
Visual Presentation:
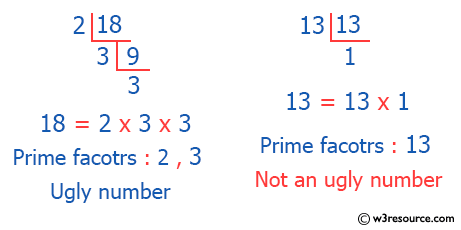
Sample Solution:
C++ Code :
# include <iostream> // Include necessary library for input and output operations
# include <string> // Include necessary library for string operations
using namespace std;
int main() {
int n, x = 0; // Declare variables n and x, initialize x to 0
cout << "\n\n Check whether a given number is an Ugly number:\n"; // Display message for user
cout << "----------------------------------------------------\n";
cout << "Input an integer number: "; // Prompt the user to input an integer number
cin >> n; // Read user input into variable n
if (n <= 0) {
cout <<"Input a correct number."; // If input is less than or equal to 0, display an error message
}
while (n != 1) { // Loop until n becomes 1
if (n % 5 == 0) {
n /= 5; // If n is divisible by 5, divide n by 5
} else if (n % 3 == 0) {
n /= 3; // If n is divisible by 3, divide n by 3
} else if (n % 2 == 0) {
n /= 2; // If n is divisible by 2, divide n by 2
} else {
cout <<"It is not an Ugly number."<<endl; // If none of the above conditions are met, it's not an ugly number
x = 1; // Set x to 1 to indicate it's not an ugly number
break; // Break out of the loop
}
}
if (x == 0) {
cout <<"It is an Ugly number."<<endl; // If x is still 0, it's an ugly number
}
return 0; // Return 0 to indicate successful completion of the program
}
Sample Output:
Check whether a given number is an Ugly number: ---------------------------------------------------- Input an integer number: 25 It is an Ugly number.
Flowchart:
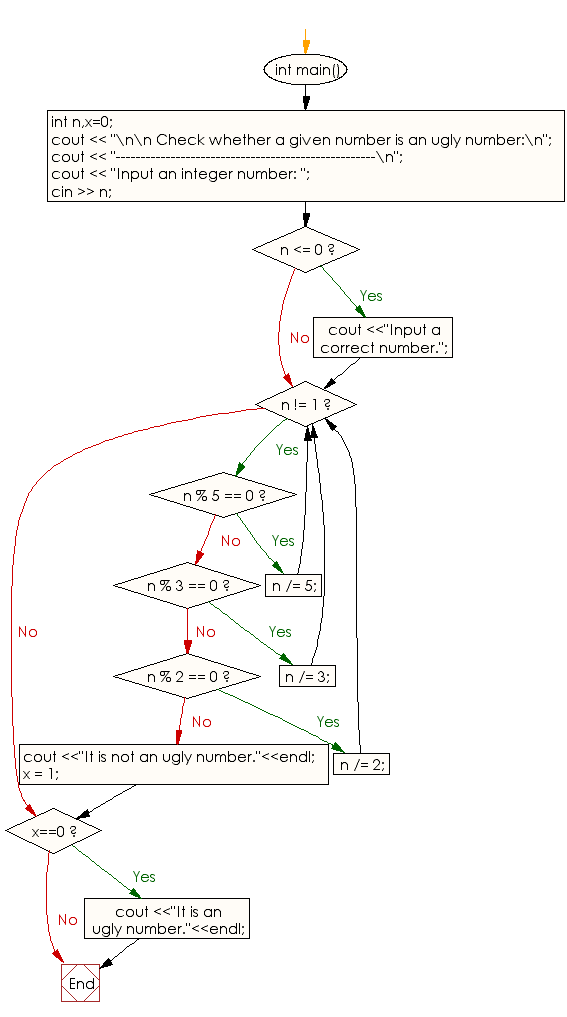
For more Practice: Solve these Related Problems:
- Write a C++ program to determine if a number is ugly by testing its divisibility only by 2, 3, and 5 using recursive division.
- Write a C++ program that verifies if a number is ugly and then outputs the factorization path that leads to 1.
- Write a C++ program to check for an ugly number without using loops—employ recursion and conditional operators instead.
- Write a C++ program to read multiple numbers and print only those that are ugly by processing an array with inline functions.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: C++ Numbers Exercises Home
Next: Write a program in C++ to check whether a given number is Abundant or not.
What is the difficulty level of this exercise?