C++ Exercises: Find the number of trailing zeroes in a given factorial
9. Count Trailing Zeroes in Factorial
Write a C++ program to find the number of trailing zeroes in a given factorial.
Input: n = 4
Output: 0
Input: n = 6
Output: 1
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to count the number of trailing zeroes in the factorial of a number
int trailing_Zeroes(int n) {
int number = 0; // Initialize the count of trailing zeroes to 0
while (n > 0) {
number += n / 5; // Count the number of multiples of 5 in n
n /= 5; // Reduce n to the next lower number for further count of trailing zeroes
}
return number; // Return the total count of trailing zeroes in the factorial of the given number
}
int main(void) {
// Test cases to find the number of trailing zeroes in factorials of different numbers
int n = 4;
cout << "\nNumber of trailing zeroes of factorial " << n << " = " << trailing_Zeroes(n) << endl;
n = 6;
cout << "\nNumber of trailing zeroes of factorial " << n << " = " << trailing_Zeroes(n) << endl;
n = 7;
cout << "\nNumber of trailing zeroes of factorial " << n << " = " << trailing_Zeroes(n) << endl;
n = 10;
cout << "\nNumber of trailing zeroes of factorial " << n << " = " << trailing_Zeroes(n) << endl;
return 0; // Return 0 to indicate successful completion
}
Sample Output:
Number of trailing zeroes of factorial 4 = 0 Number of trailing zeroes of factorial 6 = 1 Number of trailing zeroes of factorial 7 = 1 Number of trailing zeroes of factorial 10 = 2
Flowchart:
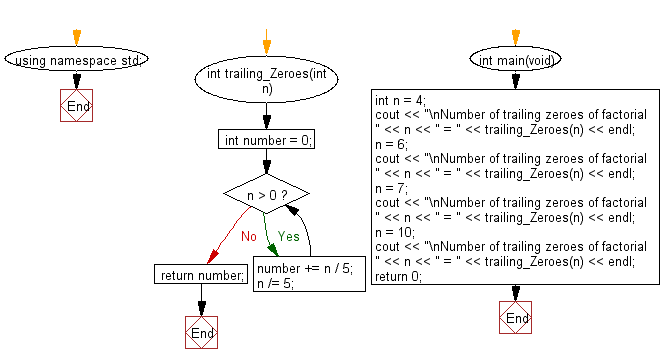
For more Practice: Solve these Related Problems:
- Write a C++ program to count the number of trailing zeroes in the factorial of a number by calculating the number of times 5 divides the factorial.
- Write a C++ program that computes the trailing zero count for n! using a loop to sum the quotients of n divided by powers of 5.
- Write a C++ program to determine the number of trailing zeroes in a factorial and then verify the result using a recursive function.
- Write a C++ program to read an integer and output the trailing zeroes in its factorial by iterating through powers of 5 until exceeding the number.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to get the column number (integer value) that corresponds to a column title as appear in an Excel sheet.
Next: Write a C++ program to count the total number of digit 1 appearing in all positive integers less than or equal to a given integer n.
What is the difficulty level of this exercise?