C++ Exercises: Get the column number that corresponds to a column title as appear in an Excel sheet
8. Excel Column Number from Title
Write a C++ program to get the column number (integer value) that corresponds to a column title as it appears on an Excel sheet.
For example:
A -> 1
B -> 2
C -> 3
...
Z -> 26
AA -> 27
AB -> 28
...
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to convert Excel column title to a corresponding number
int excel_title_to_Number(string s) {
int number = 0; // Initialize the resulting number to 0
for (const auto& c : s) { // Loop through each character in the input string
number *= 26; // Multiply the number by 26 (Excel's base)
number += c - 'A' + 1; // Convert the character to its corresponding numeric value and add to the result
}
return number; // Return the calculated number
}
int main(void) {
// Test cases to convert Excel column titles to numbers
string col_title1 = "C";
cout << "\nExcel column title = " << col_title1 << ", Corresponding number = " << excel_title_to_Number(col_title1) << endl;
col_title1 = "AD";
cout << "\nExcel column title = " << col_title1 << ", Corresponding number = " << excel_title_to_Number(col_title1) << endl;
col_title1 = "WX";
cout << "\nExcel column title = " << col_title1 << ", Corresponding number = " << excel_title_to_Number(col_title1) << endl;
return 0; // Return 0 to indicate successful completion
}
Sample Output:
Excel column title = C, Corresponding number = 3 Excel column title = AD, Corresponding number = 30 Excel column title = WX, Corresponding number = 622
Flowchart:
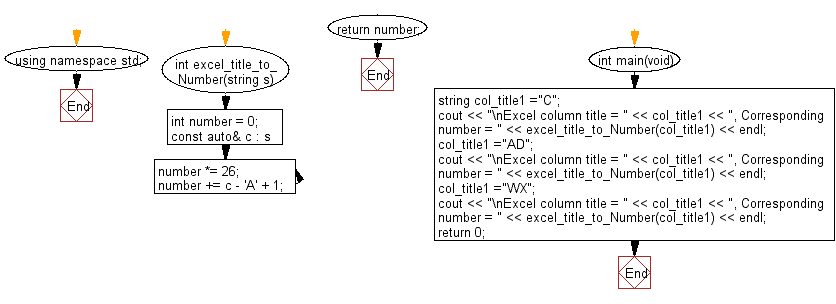
For more Practice: Solve these Related Problems:
- Write a C++ program to convert an Excel column title into its corresponding column number by processing each character and calculating its positional value.
- Write a C++ program that reads a string representing an Excel column title and computes the column number using a loop.
- Write a C++ program to implement a function that converts Excel column titles to numbers, handling both uppercase and lowercase letters.
- Write a C++ program that takes multiple Excel column titles as input and outputs their corresponding numbers in a vector.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to get the Excel column title that corresponds to a given column number (integer value).
Next: Write a C++ program to find the number of trailing zeroes in a given factorial.
What is the difficulty level of this exercise?