C++ Exercises: Check whether a given positive integer is a perfect square or not
30. Check if Number is a Perfect Square
Write a C++ program to check whether a given positive integer is a perfect square or not.
In mathematics, a square number or perfect square is an integer that is the square of an integer, in other words, it is the product of some integer with itself. For example, 9 is a square number, since it can be written as 3 × 3.
Sample Input: n = 1
Is 1 is perfect number? 1
Sample Input: n = 13
Is 13 is perfect number? 0
Sample Solution:
C++ Code :
#include <iostream>
#include <cmath>
using namespace std;
// Function to check if 'num' is a perfect square
bool is_Perfect_Square(int num) {
// Initialize start and end numbers for binary search
long long start_num = 0;
long long end_num = num;
// Binary search to find the perfect square
while(start_num + 1 < end_num) {
long long mid_num = start_num + (end_num - start_num) / 2;
if (mid_num * mid_num < num) {
start_num = mid_num; // If square of mid_num is less than 'num', update the start_num
} else if(mid_num * mid_num > num) {
end_num = mid_num; // If square of mid_num is greater than 'num', update the end_num
} else {
return true; // If mid_num is a perfect square, return true
}
}
// Check if start_num or end_num square is equal to 'num'
return start_num * start_num == num || end_num * end_num == num;
}
int main()
{
int n = 1;
// Check if 'n' is a perfect square
cout << "\nIs "<< n << " is perfect number? " << is_Perfect_Square(n) << endl;
n = 13;
// Check if 'n' is a perfect square
cout << "\nIs "<< n << " is perfect number? " << is_Perfect_Square(n) << endl;
n = 16;
// Check if 'n' is a perfect square
cout << "\nIs "<< n << " is perfect number? " << is_Perfect_Square(n) << endl;
n = 125;
// Check if 'n' is a perfect square
cout << "\nIs "<< n << " is perfect number? " << is_Perfect_Square(n) << endl;
return 0;
}
Sample Output:
Is 1 is perfect number? 1 Is 13 is perfect number? 0 Is 16 is perfect number? 1 Is 125 is perfect number? 0
Flowchart:
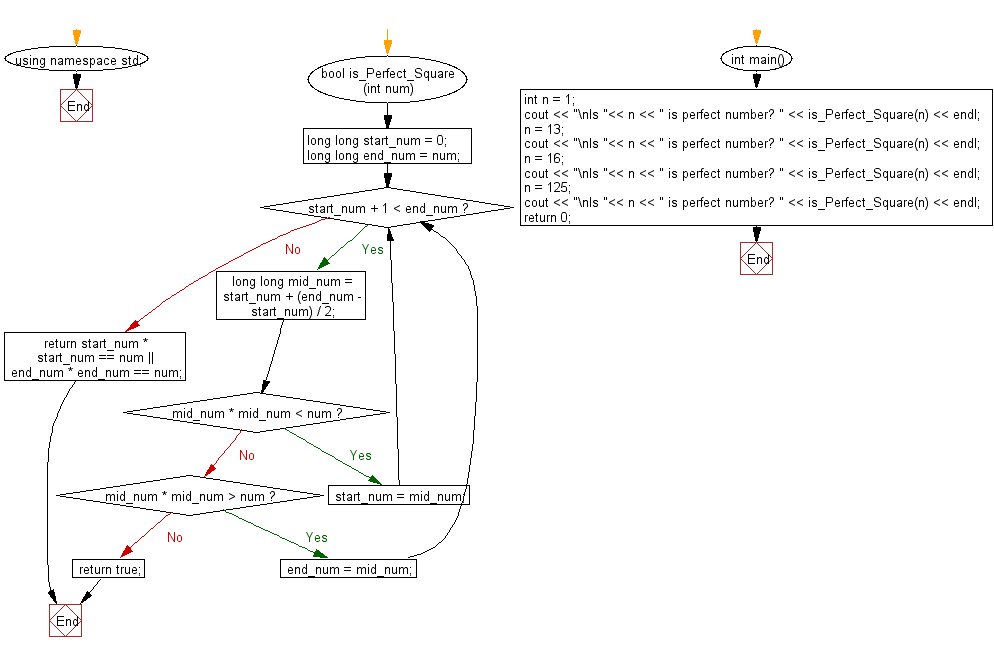
For more Practice: Solve these Related Problems:
- Write a C++ program to check whether a given number is a perfect square using integer square root approximation.
- Write a C++ program that reads an integer and verifies if it is a perfect square by comparing the square of its rounded square root.
- Write a C++ program to determine if a number is a perfect square by iterating through possible square roots until the square exceeds the number.
- Write a C++ program that uses binary search to check if an integer is a perfect square by finding the floor square root and testing its square.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program that takes a number (n) and counts all numbers with unique digits of length y within a specified range.
Next: Write a C++ program to replace a given number until it become 1.
What is the difficulty level of this exercise?