C++ Exercises: Reverse the digits of a given integer
3. Reverse Digits of an Integer
Write a C++ program to reverse the digits of a given integer.
Sample Input: 4
Sample Output: 4
Sample Input: 123
Sample Output: 321
Sample Solution:
C++ Code :
#include <iostream> // Include input/output stream library
using namespace std; // Using standard namespace
// Function to reverse an integer
int reverse_int(int x) {
int res = 0; // Initialize the result variable to store the reversed integer
// Loop to reverse the integer
while (x != 0) {
int pop = x % 10; // Extract the last digit of the number
x = x / 10; // Update the number by removing its last digit
int candidate = res * 10 + pop; // Form the reversed number
// Check for overflow by comparing the reversed number with its expected value after multiplication
if (candidate / 10 != res) {
return 0; // Return 0 if overflow occurs
}
res = candidate; // Update the result with the reversed number
}
return res; // Return the reversed integer
}
int main() {
// Test cases to reverse integers and display the results
cout << "Reverse of 4 is " << reverse_int(4) << endl;
cout << "Reverse of 123 is " << reverse_int(123) << endl;
cout << "Reverse of 208478933 is " << reverse_int(208478933) << endl;
cout << "Reverse of -73634 is " << reverse_int(-73634) << endl;
return 0; // Return 0 to indicate successful completion
}
Sample Output:
Reverse of 4 is 4 Reverse of 123 is 321 Reverse of 208478933 is 339874802 Reverse of -73634 is -43637
Flowchart:
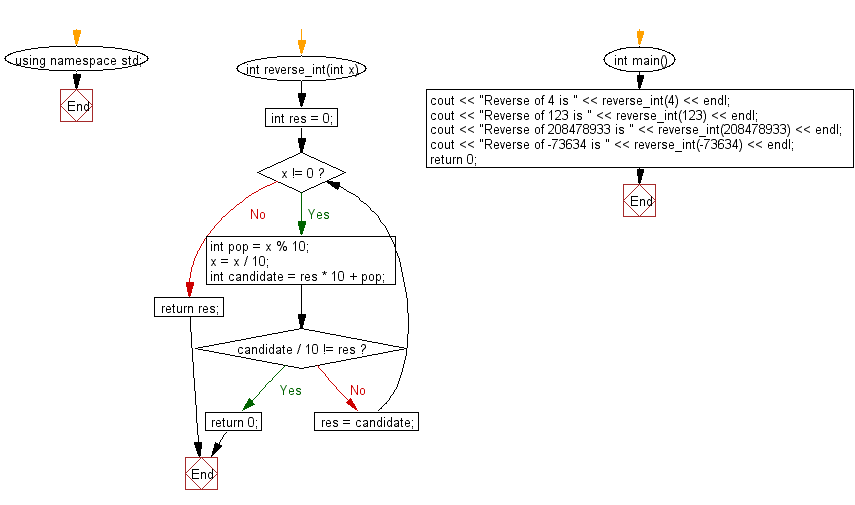
For more Practice: Solve these Related Problems:
- Write a C++ program to reverse the digits of an integer recursively and print the reversed number.
- Write a C++ program that reverses an integer by converting it to a string, then reversing the string and converting it back to an integer.
- Write a C++ program to reverse an integer without converting it to a string by using modulo and division operations in a loop.
- Write a C++ program to implement a function that reverses the digits of a number and checks if the reversed number is equal to the original (palindrome test).
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check the additive persistence of a given number.
Next: Write a C++ program to divide two integers (dividend and divisor) without using multiplication, division and mod operator.
What is the difficulty level of this exercise?