C++ Exercises: Break a given integer in at least two parts to maximize the product of those integers
28. Maximum Product from Breaking an Integer
Write a C++ program to break a given integer into at least two parts (positive integers) to maximize the product of those integers.
Sample Input: n = 5
After breaking in +ve integers maximumn product from 5 = 6
Sample Input: n = 12
After breaking in +ve integers maximumn product from 12 = 81
Sample Solution:
C++ Code :
#include <iostream>
#include <cmath>
using namespace std;
// Function to find the maximum product after breaking the integer 'n' into positive integers and multiplying them
int integer_Break(int n) {
// Base cases for 'n' equals 2 and 3
if (n == 2) {
return 1;
} else if (n == 3) {
return 2;
}
// Check if 'n' is divisible by 3
else if (n % 3 == 0) {
return (int)pow(3, n / 3); // Return the maximum product for 'n' divisible by 3
}
// Check if 'n' modulo 3 equals 1
else if (n % 3 == 1) {
return 2 * 2 * (int)pow(3, (n - 4) / 3); // Return the maximum product for 'n' modulo 3 equals 1
} else {
return 2 * (int)pow(3, n / 3); // Return the maximum product for other cases
}
}
int main() {
int n = 5; // (3 + 2) -> 3 x 2 = 6
cout << "\nAfter breaking into positive integers, the maximum product from " << n << " = " << integer_Break(n) << endl;
n = 12; // (3 + 3 + 3 + 3) -> 3 x 3 x 3 x 3 = 81
cout << "\nAfter breaking into positive integers, the maximum product from " << n << " = " << integer_Break(n) << endl;
n = 17; // (3 + 3 + 3 + 3 + 3 + 2) -> 3 x 3 x 3 x 3 x 3 x 2 = 486
cout << "\nAfter breaking into positive integers, the maximum product from " << n << " = " << integer_Break(n) << endl;
return 0;
}
Sample Output:
After breaking in +ve integers maximumn product from 5 = 6 After breaking in +ve integers maximumn product from 12 = 81 After breaking in +ve integers maximumn product from 17 = 486
Flowchart:
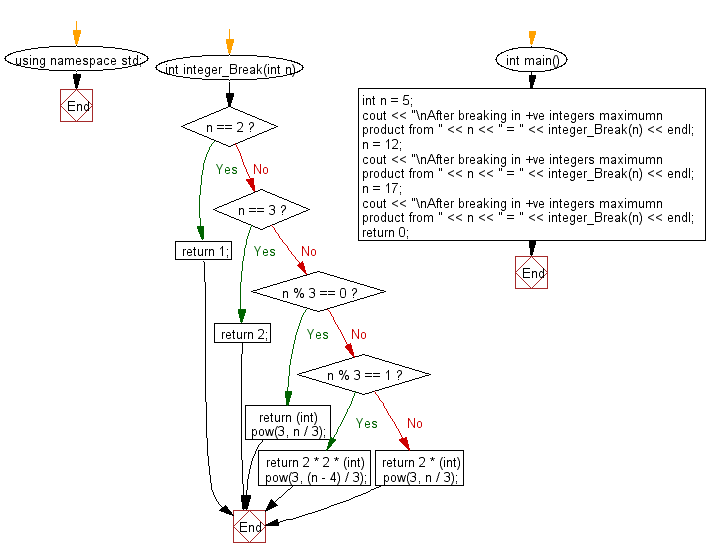
For more Practice: Solve these Related Problems:
- Write a C++ program to recursively break an integer into at least two positive integers to maximize the product, then print the maximum product.
- Write a C++ program that uses dynamic programming to find the maximum product obtainable by partitioning a number.
- Write a C++ program to compare the maximum product obtained by breaking an integer into equal parts versus unequal parts.
- Write a C++ program that reads an integer and outputs the optimal break-down yielding the maximum product along with the product value.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to find the number of perfect square (e.g. 1, 4, 9, 16, ...) numbers which represent a sum of a given number.
Next: Write a C++ program that takes a number (n) and counts all numbers with unique digits of length y within a specified range.
What is the difficulty level of this exercise?