C++ Exercises: Count the prime numbers less than a given positive number
Write a C++ program to count prime numbers less than a given positive number.
Sample Input: n = 8
Sample Output: Number of prime numbers less than 8 is 2
Sample Input: n = 30
Sample Output: Number of prime numbers less than 30 is 10
Sample Solution:
C++ Code :
#include <iostream>
#include <cmath>
using namespace std;
class Solution {
public:
// Function to count prime numbers less than 'n'
int count_Primes(int n) {
int ctr = 0; // Initialize counter for prime numbers
for (int i = 2; i < n; i++) {
if (is_Prime(i)) { // Check if 'i' is a prime number
ctr++; // Increment counter if 'i' is prime
}
}
return ctr; // Return the count of prime numbers
}
// Function to check if a number is prime
bool is_Prime(int n) {
int n_ = int(sqrt(n)); // Compute square root of 'n'
for (int i = 2; i <= n_; i++) {
if (0 == n % i) { // Check if 'n' is divisible by 'i'
return false; // If divisible, 'n' is not a prime number
}
}
return true; // If 'n' is not divisible by any 'i', 'n' is a prime number
}
};
int main() {
Solution *solution = new Solution(); // Create an instance of Solution class
int n = 8;
// Display the number of prime numbers less than 'n'
cout << "Number of prime numbers less than " << n << " is " << solution->count_Primes(5) << endl;
n = 30;
cout << "Number of prime numbers less than " << n << " is " << solution->count_Primes(30) << endl;
n = 100;
cout << "Number of prime numbers less than " << n << " is " << solution->count_Primes(100) << endl;
return 0;
}
Sample Output:
Number of prime numbers less than 8 is 2 Number of prime numbers less than 30 is 10 Number of prime numbers less than 100 is 25
Flowchart:
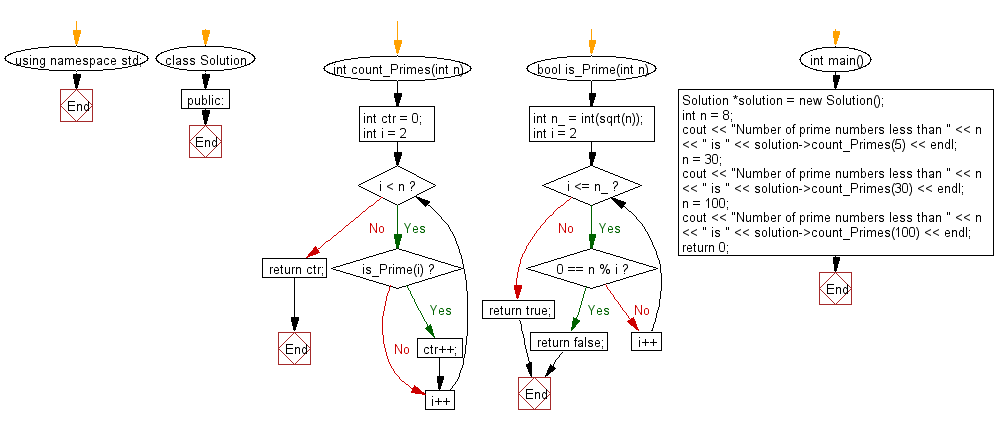
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to compute square root of a given non-negative integer.
Next: Write a C++ program to count the total number of digit 1 pressent in all positive numbers less than or equal to a given integer.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics