C++ Exercises: Compute square root of a given non-negative integer
Write a C++ program to compute the square root of a given non-negative integer. Return type should be an integer.
Sample Input: n = 81
Sample Output: Square root of 81 = 9
Sample Input: n = 8
Sample Output: Square root of 8 = 2
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to find the square root of a number using binary search
int square_root(int num1) {
long left_part = 0; // Initializing the left boundary
long right_part = num1 / 2 + 1; // Initializing the right boundary
// Implementing binary search to find the square root
while (left_part <= right_part) {
long mid = left_part + (right_part - left_part) / 2; // Calculating the midpoint
long result = mid * mid; // Calculating the square of the midpoint
// Checking conditions to adjust the boundaries and find the square root
if (result == (long)num1) {
return (int)mid; // If square of mid equals the number, return mid as square root
} else if (result > num1) {
right_part = mid - 1; // If square of mid is greater, adjust the right boundary
} else {
left_part = mid + 1; // If square of mid is smaller, adjust the left boundary
}
}
return (int)right_part; // Returning right_part as square root
}
// Main function to test the square_root function
int main() {
int n = 81;
cout << "\nSquare root of " << n << " = " << square_root(n) << endl; // Testing square root of 81
n = 8;
cout << "\nSquare root of " << n << " = " << square_root(n) << endl; // Testing square root of 8
n = 627;
cout << "\nSquare root of " << n << " = " << square_root(n) << endl; // Testing square root of 627
n = 225;
cout << "\nSquare root of " << n << " = " << square_root(n) << endl; // Testing square root of 225
return 0;
}
Sample Output:
Square root of 81 = 9 Square root of 8 = 2 Square root of 627 = 25 Square root of 225 = 15
Flowchart:
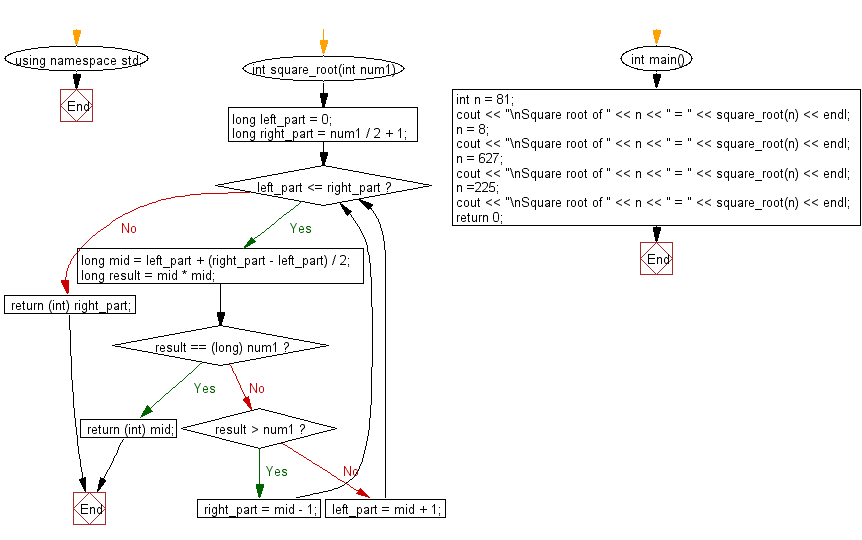
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to compute the sum of two given binary strings.
Next: Write a C++ program to count the prime numbers less than a given positive number.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics