C++ Exercises: Compute the sum of two given binary strings
22. Sum of Two Binary Strings
Write a C++ program to compute the sum of a pair of binary strings. Binary strings will be returned, and input strings shouldn't be blank or contain just 1 or 0 characters.
Sample Input: bstr1 = "10"
bstr2 = "1"
Sample Output: 10 + 1 = 11
Sample Input: bstr1 = "1100"
bstr2 = "1010"
Sample Output: 1100 + 1010 = 10110
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to add two binary strings
string binary_add(string bstr1, string bstr2) {
// Determine the size of the result string
int size = max(bstr1.size(), bstr2.size());
int temp = 0;
// Initialize an empty string to store the result
string result_str = "";
// Loop through each position of the binary strings and perform addition
for (auto i = 0; i < size; ++i) {
// Extract individual digits of binary strings
int digit1 = (i + 1 <= bstr1.size()) ? bstr1[bstr1.size() - 1 - i] - '0' : 0;
int digit2 = (i + 1 <= bstr2.size()) ? bstr2[bstr2.size() - 1 - i] - '0' : 0;
// Calculate the sum of digits along with the carry
int number = (digit1 + digit2 + temp);
temp = number / 2;
// Append the sum digit to the result string
result_str = to_string(number % 2) + result_str;
}
// If there's a remaining carry, add it to the result
if (temp > 0) {
result_str = to_string(temp) + result_str;
}
return result_str; // Return the final result
}
// Main function to test binary_add function
int main() {
string bstr1 = "10";
string bstr2 = "1";
cout << "\n" << bstr1 << " + " << bstr2 << " = " << binary_add(bstr1, bstr2) << endl;
bstr1 = "1100";
bstr2 = "1010";
cout << "\n" << bstr1 << " + " << bstr2 << " = " << binary_add(bstr1, bstr2) << endl;
bstr1 = "10";
bstr2 = "10";
cout << "\n" << bstr1 << " + " << bstr2 << " = " << binary_add(bstr1, bstr2) << endl;
return 0;
}
Sample Output:
10 + 1 = 11 1100 + 1010 = 10110 10 + 10 = 100
Flowchart:
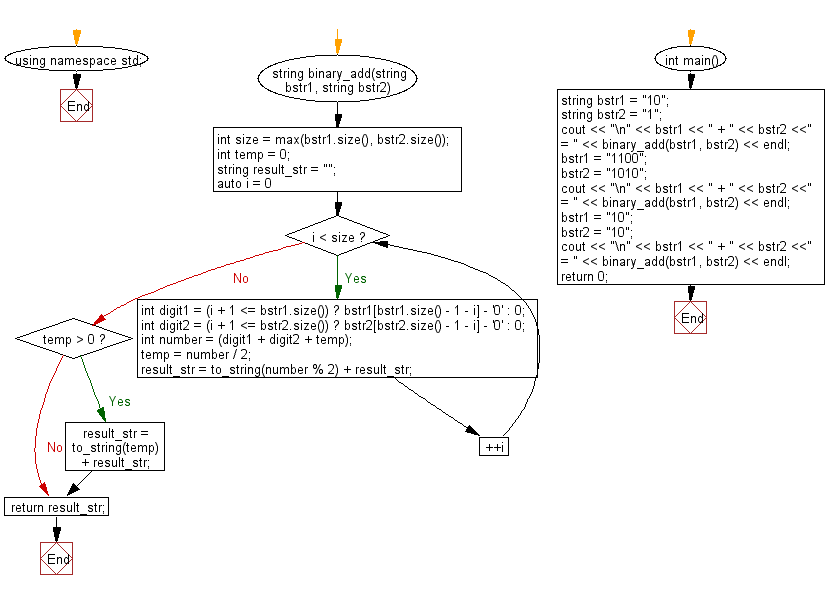
For more Practice: Solve these Related Problems:
- Write a C++ program to add two binary strings without converting them to integers, by simulating binary addition.
- Write a C++ program that processes two binary strings and outputs their sum by iterating from the least significant bit.
- Write a C++ program to implement binary string addition using recursion to handle the carry propagation.
- Write a C++ program that reads two binary strings, aligns them by padding zeros, and then performs digit-by-digit addition to output the result.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check if a given string is a decimal number or not.
Next: Write a C++ program to compute square root of a given non-negative integer.What is the difficulty level of this exercise?