C++ Exercises: Check if a given string is a decimal number or not
21. Check if a String is a Decimal Number
A decimal number is defined as a number whose whole number and fractional parts are separated by a decimal point in algebra. Write a C++ program to check if a given string is a decimal number or not.
List of characters of a valid decimal number:
Numbers: 0-9
Positive/negative sign - "+"/"-"
Decimal point - "."
Exponent - "e"
Sample Input: s = 9
Sample Output: Is 0 a decimal number? 1
Sample Input: s = abc 123
Sample Output: Is abc 123 a decimal number? 0
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to check if the given string is a decimal number or not
bool isNumber(string str_num) {
int i = 0;
int str_len = str_num.size();
// Skipping leading spaces
while (i < str_len && str_num[i] == ' ')
i++;
// Handling sign (+/-)
if (i < str_len && (str_num[i] == '+' || str_num[i] == '-'))
i++;
int digits = 0, dots = 0;
// Loop to check for digits and dots in the string
while (i < str_len && ((str_num[i] >= '0' && str_num[i] <= '9') || (str_num[i] == '.'))) {
if (str_num[i] >= '0' && str_num[i] <= '9')
digits++;
else if (str_num[i] == '.')
dots++;
i++;
}
// Conditions for an invalid number
if (digits == 0 || dots > 1)
return false;
// Handling exponential notation (e)
if (i < str_len && str_num[i] == 'e') {
// Handling sign (+/-) after 'e'
if (++i < str_len && (str_num[i] == '+' || str_num[i] == '-'))
i++;
// Checking for valid exponential notation
if (i == str_len || (str_num[i] < '0' || str_num[i] > '9'))
return false;
// Skipping exponential part
while (i < str_len && (str_num[i] >= '0' && str_num[i] <= '9'))
i++;
}
// Skipping optional trailing spaces
while (i < str_len && str_num[i] == ' ')
i++;
return (i == str_len); // If at the end of the string, then it's a valid number
}
// Main function to test isNumber() function
int main() {
string s = "0";
cout << "\n Is " << s << " a decimal number? " << isNumber(s) << endl;
s = "abc 123";
cout << "\n Is " << s << " a decimal number? " << isNumber(s) << endl;
s = "abc";
cout << "\n Is " << s << " a decimal number? " << isNumber(s);
s = "0.12";
cout << "\n Is " << s << " a decimal number? " << isNumber(s) << endl;
s = "123.33";
cout << "\n Is " << s << " a decimal number? " << isNumber(s) << endl;
s = "76.4e93";
cout << "\n Is " << s << " a decimal number? " << isNumber(s) << endl;
s = "+123";
cout << "\n Is " << s << " a decimal number? " << isNumber(s) << endl;
s = "+-33";
cout << "\n Is " << s << " a decimal number? " << isNumber(s) << endl;
return 0;
}
Sample Output:
Is 0 a decimal number? 1 Is abc 123 a decimal number? 0 Is abc a decimal number? 0 Is 0.12 a decimal number? 1 Is 123.33 a decimal number? 1 Is 76.4e93 a decimal number? 1 Is +123 a decimal number? 1 Is +-33 a decimal number? 0
Flowchart:
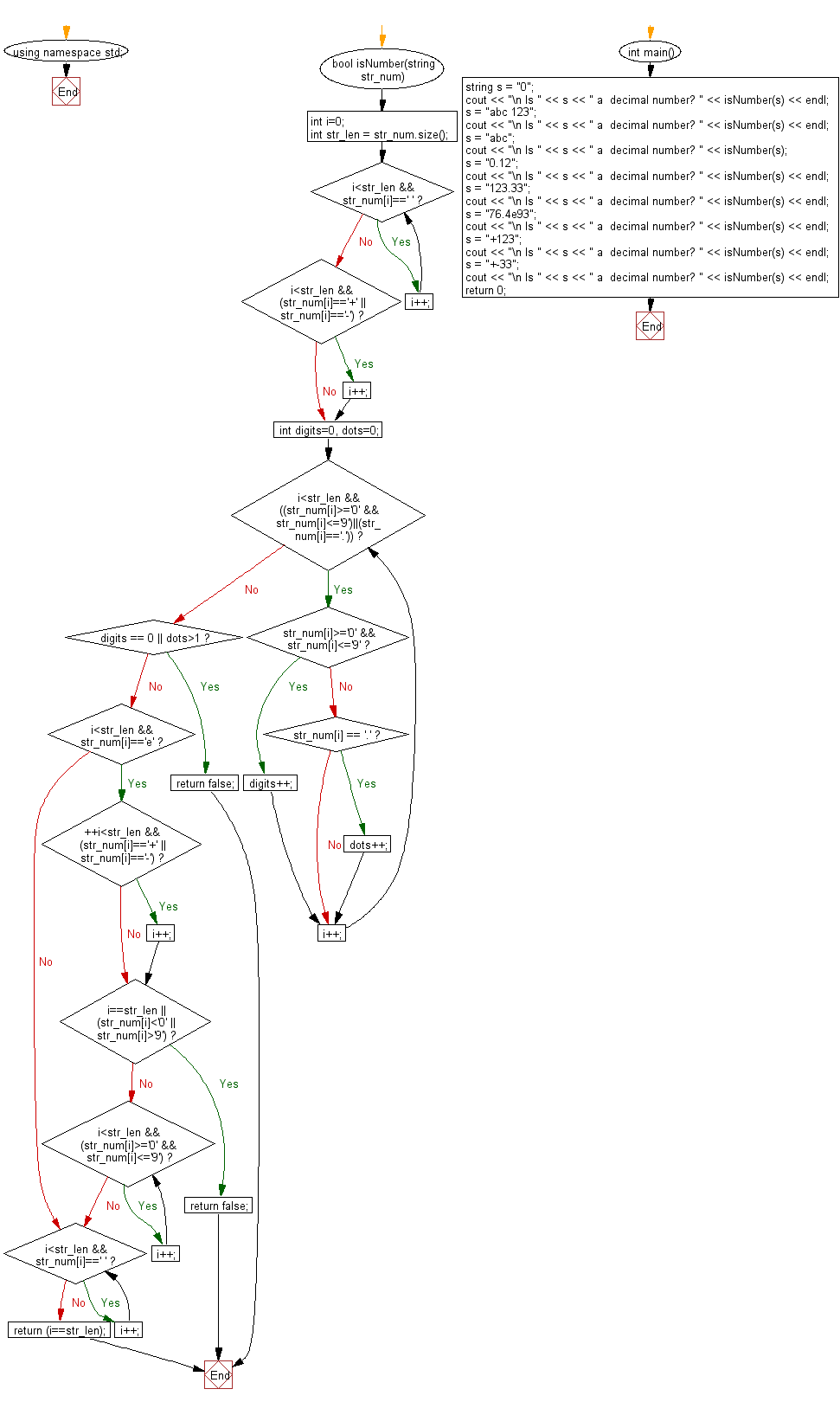
For more Practice: Solve these Related Problems:
- Write a C++ program to validate whether an input string represents a valid decimal number, considering optional sign and exponent.
- Write a C++ program that uses regular expressions to check if a string is a properly formatted decimal number.
- Write a C++ program to parse a string character by character and determine if it is a valid decimal number with optional decimals and exponents.
- Write a C++ program that reads a string and returns true if it is a valid decimal number, using state machines to process the input.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to calculate the product of two positive integers represented as strings.
Next: Write a C++ program to compute the sum of two given binary strings. Return result will be a binary string and input strings should not be blank and contains only 1 or 0 charcters.What is the difficulty level of this exercise?