C++ Exercises: Calculate the product of two positive integers represented as strings
20. Multiply Two Integers Represented as Strings
Write a C++ program to calculate the product of two positive integers represented as strings. Return the result as a string.
Sample Input: sn1 = "12"
sn2 = "5"
Sample Output: 12 X 5 = 60
Sample Input: sn1 = "48"
sn2 = "85"
Sample Output: 48 X 85 = 4080
Sample Solution:
C++ Code :
#include <iostream>
#include <algorithm>
#include <vector>
#include <functional>
using namespace std;
// Function to multiply two numbers represented as strings
string multiply(string sn1, string sn2) {
// Lambda function to convert character to integer value
const auto char_to_int = [](const char c) { return c - '0'; };
// Lambda function to convert integer to character value
const auto int_to_char = [](const int i) { return i + '0'; };
// Convert string representation of numbers to integer vectors in reverse order
vector<int> n1;
transform(sn1.rbegin(), sn1.rend(), back_inserter(n1), char_to_int);
vector<int> n2;
transform(sn2.rbegin(), sn2.rend(), back_inserter(n2), char_to_int);
// Create a temporary vector to store intermediate multiplication results
vector<int> temp(n1.size() + n2.size());
// Perform multiplication of numbers digit by digit
for(int i = 0; i < n1.size(); ++i) {
for(int j = 0; j < n2.size(); ++j) {
temp[i + j] += n1[i] * n2[j];
temp[i + j + 1] += temp[i + j] / 10;
temp[i + j] %= 10;
}
}
// Convert the temporary vector to string representation of the result
string result;
transform(find_if(temp.rbegin(), prev(temp.rend()),
[](const int i) { return i != 0; }),
temp.rend(), back_inserter(result), int_to_char);
return result;
}
// Main function to test the multiply function
int main()
{
// Test cases for multiplying two numbers represented as strings
string sn1 = "12";
string sn2 = "5";
cout << sn1 <<" X " << sn2 << " = " << multiply(sn1, sn2) << endl;
sn1 = "48";
sn2 = "85";
cout << sn1 <<" X " << sn2 << " = " << multiply(sn1, sn2) << endl;
return 0;
}
Sample Output:
12 X 5 = 60 48 X 85 = 4080
Flowchart:
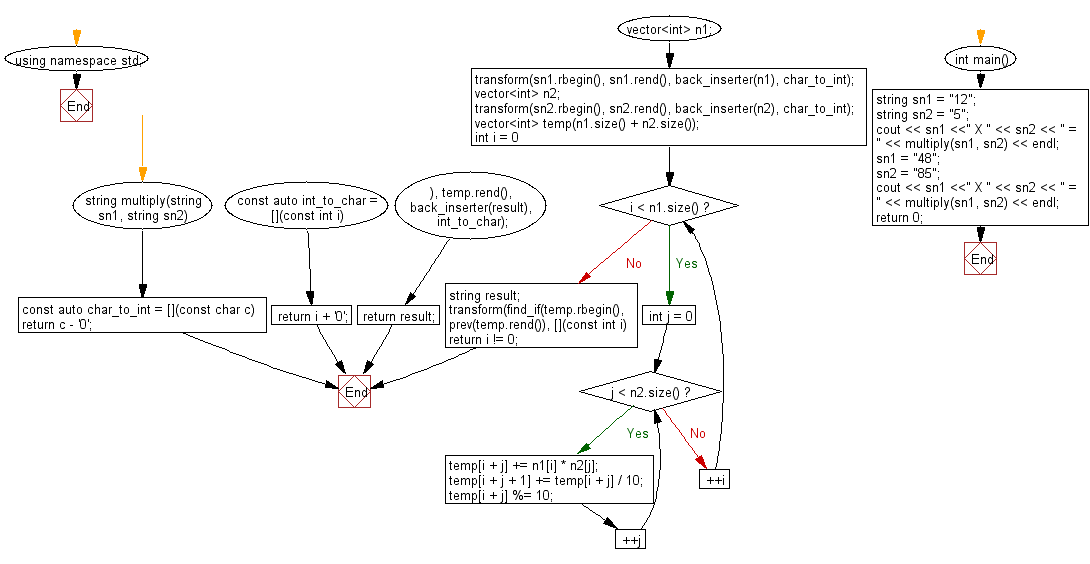
For more Practice: Solve these Related Problems:
- Write a C++ program to multiply two large numbers given as strings using elementary multiplication without built-in big integer libraries.
- Write a C++ program that implements string multiplication by simulating the manual multiplication algorithm and returns the result as a string.
- Write a C++ program to perform multiplication on two numeric strings and handle cases with leading zeros appropriately.
- Write a C++ program that multiplies two integers represented as strings by converting them to arrays of digits and performing digit-wise multiplication.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to convert a given roman numeral to a integer.
Next: Write a C++ program to check if a given string is a decimal number or not.What is the difficulty level of this exercise?