C++ Exercises: Check the additive persistence of a given number
2. Additive Persistence and Digital Root
Write a C++ program to check the additive persistence of a given number.
Additive Persistence
Consider the process of taking a number, adding its digits, then adding the digits of the number derived from it, etc., until the remaining number has only one digit. The number of additions required to obtain a single digit from a number n is called the additive persistence of n, and the digit obtained is called the digital root of n.
For example, the sequence obtained from the starting number 9876 is (9876, 30, 3), so 9876 has an additive persistence of 2 and a digital root of 3. The additive persistences of the first few positive integers are 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 2, 1, ... (OEIS A031286). The smallest numbers of additive persistence n for n=0, 1, ... are 0, 10, 19, 199, 19999999999999999999999, ... (OEIS A006050).
Source: https://mathworld.wolfram.com/
Sample Solution:
C++ Code :
#include <iostream> // Include input/output stream library
#include <string> // Include string manipulation library
#include <sstream> // Include stringstream for string conversions
using namespace std; // Using standard namespace
// Function to calculate the additive persistence of a number
int AdditivePersistence(int num) {
int ctr = 0; // Counter to track the number of iterations
// If the number is a single digit, return 0 as additive persistence
if (num < 10)
{
return 0;
}
stringstream convert; // Create a stringstream object for conversion
convert << num; // Insert the number into the stringstream
string y = convert.str(); // Convert the number to a string
int total_num, n; // Variables for calculations
// Loop until the total is reduced to a single digit
do
{
total_num = 0; // Initialize the total sum to 0
ctr++; // Increment the counter for each iteration
// Calculate the sum of individual digits in the number
for (int x = 0; x < y.length(); x++)
{
n = int(y[x]) - 48; // Convert character to integer by ASCII conversion
total_num += n; // Add the digit to the total sum
}
stringstream convert; // Create a stringstream object for conversion
convert << total_num; // Insert the total sum into the stringstream
y = convert.str(); // Convert the total sum to a string
} while (total_num >= 10); // Repeat until the total sum is a single digit
return ctr; // Return the number of iterations
}
int main() {
// Test cases to calculate additive persistence
cout << "Additive persistence of 4 is " << AdditivePersistence(4) << endl;
cout << "\nAdditive persistence of 125 is " << AdditivePersistence(125) << endl;
cout << "\nAdditive persistence of 5489 is " << AdditivePersistence(5489) << endl;
cout << "\nAdditive persistence of 36024 is " << AdditivePersistence(36024) << endl;
return 0; // Return 0 to indicate successful completion
}
Sample Output:
Additive persistence of 4 is 0 Additive persistence of 125 is 1 Additive persistence of 5489 is 2 Additive persistence of 36024 is 2
Flowchart:
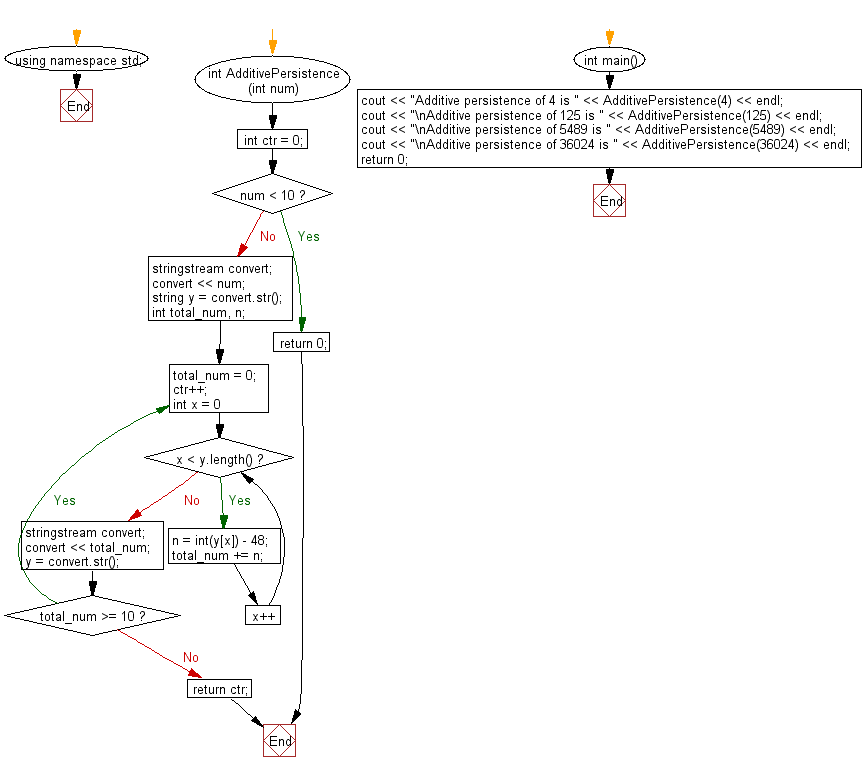
For more Practice: Solve these Related Problems:
- Write a C++ program to recursively compute the additive persistence of a number and output both the persistence and its digital root.
- Write a C++ program that calculates the additive persistence by iteratively summing the digits until a single digit remains, and then prints the number of iterations.
- Write a C++ program to implement a function that uses recursion to determine the digital root of a number and counts the number of recursive calls.
- Write a C++ program that reads a number, computes its additive persistence using a while loop, and compares the result with a recursive implementation.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to check whether a given number is a power of two or not.
Next: Write a C++ program to reverse the digits of a given integer.
What is the difficulty level of this exercise?