C++ Exercises: Convert a given roman numeral to a integer
Write a C++ program to convert a given integer to a Roman numeral.
Sample Input: n = VII
Sample Output: Integer 7
Sample Input: n = XIX
Sample Output: Integer 19
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to convert a Roman numeral to an integer
int roman_to_integer(string rstr) {
int n = int(rstr.length());
if (0 == n) {
return 0;
}
// Initialize the result
int result = 0;
// Iterate through each character in the Roman numeral string
for (int i = 0; i < n; i++) {
// Switch statement to handle different Roman numeral characters
switch (rstr[i]) {
case 'I':
result += 1;
break;
case 'V':
result += 5;
break;
case 'X':
result += 10;
break;
case 'L':
result += 50;
break;
case 'C':
result += 100;
break;
case 'D':
result += 500;
break;
case 'M':
result += 1000;
break;
}
}
// Loop to handle subtraction cases (like IV, IX, XL, XC, etc.)
for (int i = 1; i < n; i++) {
if ((rstr[i] == 'V' || rstr[i] == 'X') && rstr[i - 1] == 'I') {
result -= 1 + 1; // Subtract 1 for I and add 1 for V or X
}
if ((rstr[i] == 'L' || rstr[i] == 'C') && rstr[i - 1] == 'X') {
result -= 10 + 10; // Subtract 10 for X and add 10 for L or C
}
if ((rstr[i] == 'D' || rstr[i] == 'M') && rstr[i - 1] == 'C') {
result -= 100 + 100; // Subtract 100 for C and add 100 for D or M
}
}
return result; // Return the resulting integer
}
// Main function to test the roman_to_integer function
int main()
{
// Test cases to convert Roman numerals to integers using the roman_to_integer function
string s = "VII";
cout << "Roman " << s << " -> Integer " << roman_to_integer(s) << endl;
s = "XIX";
cout << "Roman " << s << " -> Integer " << roman_to_integer(s) << endl;
s = "DCCLXXXIX";
cout << "Roman " << s << " -> Integer " << roman_to_integer(s) << endl;
s = "MXCIX";
cout << "Roman " << s << " -> Integer " << roman_to_integer(s) << endl;
s = "MMMMMMMMMMMMMMMMMMMMMMMCDLVI";
cout << "Roman " << s << " -> Integer " << roman_to_integer(s) << endl;
return 0;
}
Sample Output:
Roman VII -> Integer 7 Roman XIX -> Integer 19 Roman DCCLXXXIX -> Integer 789 Roman MXCIX -> Integer 1099 Roman MMMMMMMMMMMMMMMMMMMMMMMCDLVI -> Integer 23456
Flowchart:
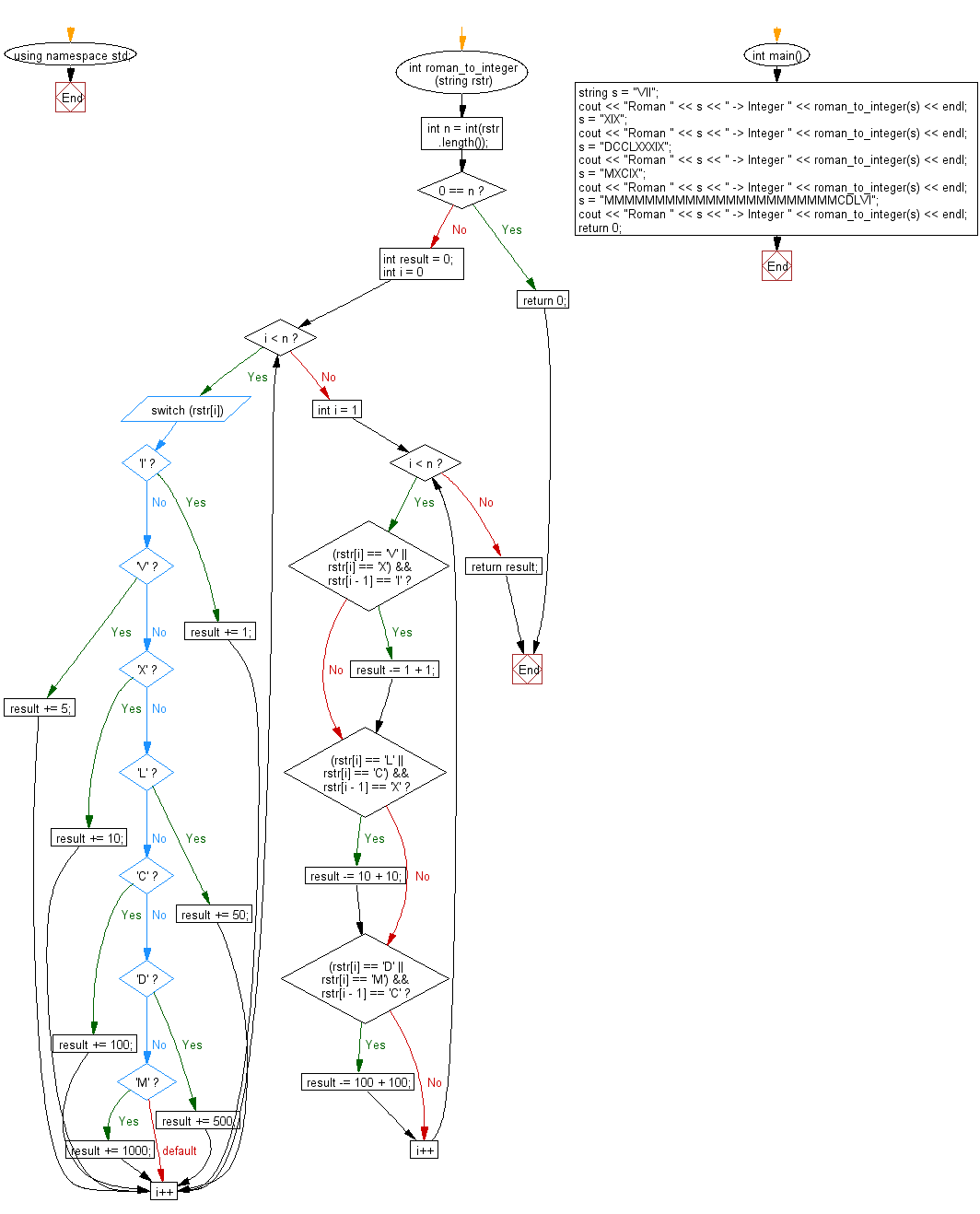
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to convert a given integer to a roman numeral.
Next: Write a C++ program to calculate the product of two positive integers represented as strings.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics