C++ Exercises: Multiply two integers without using multiplication, division, bitwise operators, and loops
17. Multiply Two Integers Without Multiplication, Division, Bitwise Operators, and Loops
Write a C++ program to multiply two integers without using multiplication, division, bitwise operators, and loops.
Sample Input: 8, 9
Sample Output: 72
Sample Input: -11, 19
Sample Output: -209
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to multiply two integers recursively
int multiply_two_nums(int x, int y)
{
// Base case: if y is 0, the result is 0
if (y == 0)
return 0;
// If y is positive, recursively add x y times
if (y > 0)
return (x + multiply_two_nums(x, y - 1));
// If y is negative, convert it to positive, multiply, and make the result negative
if (y < 0)
return -multiply_two_nums(x, -y);
}
int main()
{
// Test cases to multiply two numbers using the multiply_two_nums function
cout << multiply_two_nums(8, 9) << endl; // Output the product of 8 and 9
cout << multiply_two_nums(-11, 19) << endl; // Output the product of -11 and 19
return 0; // Return 0 to indicate successful completion
}
Sample Output:
72 -209
Flowchart:
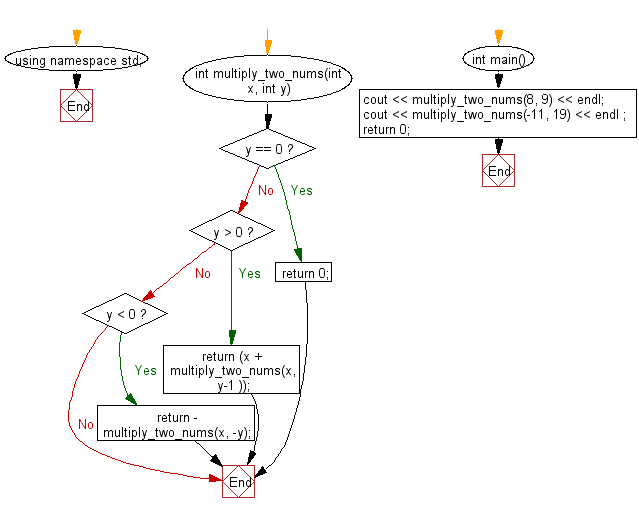
For more Practice: Solve these Related Problems:
- Write a C++ program to recursively multiply two integers using repeated addition and subtraction without loops.
- Write a C++ program that uses recursion and conditional statements to multiply two numbers without using multiplication or bitwise operators.
- Write a C++ program to multiply two integers by simulating the multiplication process recursively, ensuring proper handling of negative numbers.
- Write a C++ program to implement a recursive function that multiplies two numbers using only addition and subtraction operations.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ programming to find the nth digit of number 1 to n.
Next: Write a C++ program to convert a given integer to a roman numeral.
What is the difficulty level of this exercise?