C++ Exercises: Find the square root of a number using Babylonian method
Write a C++ program to find the square root of a number using the Babylonian method.
Sample Input: n = 50
Sample Output: 7.07107
Sample Input: n = 81
Sample Output: 9
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to find the square root of a number
float square_Root(float num)
{
float x = num; // Initializing x as the given number
float y = 1; // Initializing y as 1 to be used in calculations
float e = 0.000001; // The desired precision of the result
// Loop to iteratively calculate the square root using the Babylonian method
while (x - y > e) {
x = (x + y) / 2; // Update x with the average of x and y
y = num / x; // Update y to be the number divided by the updated x
}
return x; // Return the calculated square root
}
int main()
{
// Testing the square_Root function for different values
int n = 50;
cout << "Square root of " << n << " is " << square_Root(n); // Display the square root of 50
n = 81;
cout << "\nSquare root of " << n << " is " << square_Root(n); // Display the square root of 81
return 0; // Return 0 to indicate successful completion
}
Sample Output:
Square root of 50 is 7.07107 Square root of 81 is 9
Flowchart:
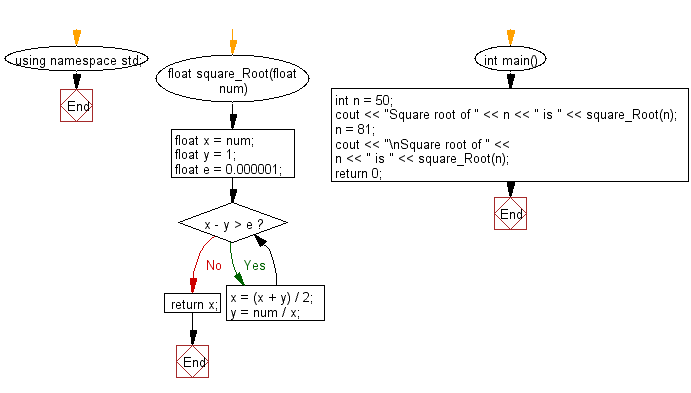
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ programming to find the nth digit of number 1 to n.
Next: Write a C++ program to multiply two integers without using multiplication, division, bitwise operators, and loops.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics