C++ Exercises: Find the nth digit of number 1 to n
Write a C++ program to find the nth digit of the number 1 to n?
Infinite integer sequence: 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12 .. where n is a positive integer.
Input: 7
Output: 7
Input: 12
Output: 1
The 12th digit of the sequence 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, ... is 1, which is part of the number 11.
Sample Solution:
C++ Code :
#include <iostream>
#include <cmath>
using namespace std;
// Function to find the nth digit of an infinite integer sequence
int find_Nth_Digit(int n) {
int digit_length = 1;
// Loop to determine the length of the digit where the nth digit exists
while (n > digit_length * 9 * pow(10, digit_length - 1)) {
n -= digit_length * 9 * pow(10, digit_length - 1);
++digit_length;
}
// Calculate the specific number containing the nth digit
const int num = pow(10, digit_length - 1) + (n - 1) / digit_length;
// Calculate the actual nth digit of the number
int result = num / pow(10, (digit_length - 1) - (n - 1) % digit_length);
result %= 10; // Obtain the last digit of the calculated number
return result;
}
int main() {
// Testing the find_Nth_Digit function for different values of n
int n = 7;
cout << "\n " << n << "th digit of the infinite integer sequence is " << find_Nth_Digit(n) << endl;
n = 13;
cout << "\n " << n << "th digit of the infinite integer sequence is " << find_Nth_Digit(n) << endl;
return 0;
}
Sample Output:
7th digit of the infinite integer sequence is 7 13th digit of the infinite integer sequence is 1
Flowchart:
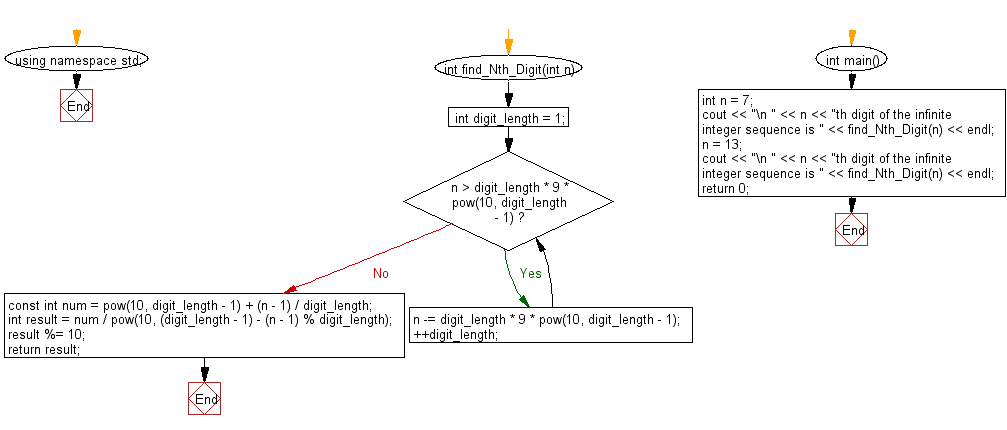
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ programming to get the maximum product from a given integer after breaking the integer into the sum of at least two positive integers.
Next: Write a C++ program to find the square root of a number using Babylonian method.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics