C++ Exercises: Check if a given integer is a power of three or not
Write a C++ program to check if a given integer is a power of three or not.
Input: 9
Output: true
Input: 81
Output: true
Input: 45
Output: false
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to check if a number is a power of three
string is_PowerOf_Three(int n) {
// Continuously divide 'n' by 3 until it's not divisible by 3
while (n % 3 == 0) {
n = n / 3;
}
// If the final value of 'n' is 1, then it is a power of three
if (1 == n) {
return "True";
} else {
return "False"; // Otherwise, it is not a power of three
}
}
int main(void) {
// Test cases to check if the numbers are powers of three
int n = 15;
cout << "\nIf " << n << " is power of three? " << is_PowerOf_Three(n) << endl;
n = 9;
cout << "\nIf " << n << " is power of three? " << is_PowerOf_Three(n) << endl;
n = 243;
cout << "\nIf " << n << " is power of three? " << is_PowerOf_Three(n) << endl;
return 0; // Return 0 to indicate successful completion
}
Sample Output:
If 15 is power of three? False If 9 is power of three? True If 243 is power of three? True
Flowchart:
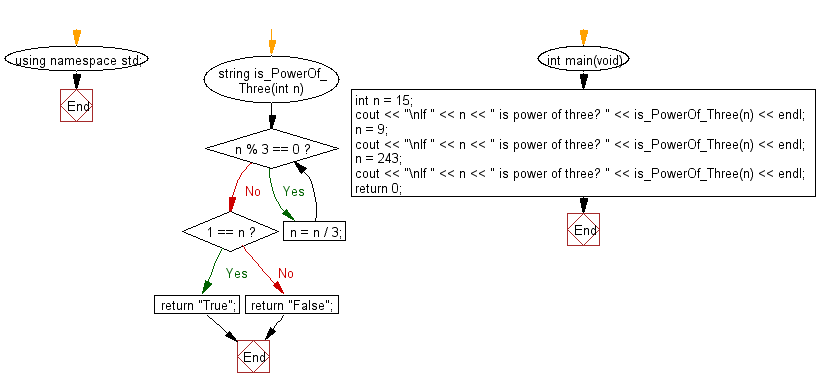
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ programming to add repeatedly all digits of a given non-negative number until the result has only one digit.
Next: For a non negative integer in the range 0 ≤ i ≤ n write a C++ programming to calculate the number of 1's in their binary representation and return them as an array.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics