C++ Exercises: Add repeatedly all digits of a given non-negative number until the result has only one digit
11. Add Digits Until Single Digit (Digital Root)
Write a C++ program to add repeatedly all digits of a given non-negative number until the result has only one digit.
Input: 58
Output: 4
Explanation: The formula is like: 5 + 8 = 13, 1 + 3 = 4.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to find the sum of digits of a number until a single digit is obtained
int addDigits(int num) {
// Formula to get the sum of digits until a single digit is achieved
return num - (num - 1) / 9 * 9;
}
int main(void) {
// Test cases to find the single digit sum of numbers
int n = 15;
cout << "\nInitial number is " << n << " single digit number is " << addDigits(n) << endl;
n = 57;
cout << "\nInitial number is " << n << " single digit number is " << addDigits(n) << endl;
return 0; // Return 0 to indicate successful completion
}
Sample Output:
Initial number is 15 single digit number is 6 Initial number is 57 single digit number is 3
Flowchart:
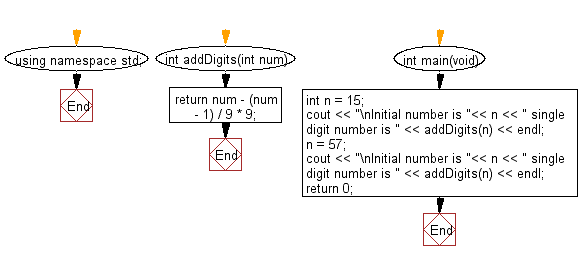
For more Practice: Solve these Related Problems:
- Write a C++ program to recursively sum the digits of a number until a single-digit result is obtained and print the digital root.
- Write a C++ program to calculate the digital root of a number using a loop and then compare the result with a mathematical formula.
- Write a C++ program that implements a recursive function to add the digits of an integer until a single digit remains, then output the number of iterations.
- Write a C++ program to read a number and repeatedly sum its digits until a one-digit number is produced, then display the process.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to count the total number of digit 1 appearing in all positive integers less than or equal to a given integer n.
Next: Write a C++ programming to check if a given integer is a power of three or not.
What is the difficulty level of this exercise?