C++ Linked List Exercises: Delete first node of a Singly Linked List
10. Delete First Node of Singly Linked List
Write a C++ program to delete first node of a given Singly Linked List.
Test Data:
Original Linked list:
13 11 9 7 5 3 1
Delete first node of Singly Linked List:
11 9 7 5 3 1
Sample Solution:
C++ Code:
#include <iostream> // Including input-output stream header file
using namespace std; // Using standard namespace
// Declare a structure for defining a Node
struct Node {
int num; // Data field to store a number
Node *next; // Pointer to the next node
};
struct Node *head = NULL; // Starting (Head) node initialized as NULL
// Function to insert a node at the start of the linked list
void insert_Node(int n) {
struct Node *new_node = new Node; // Creating a new node
new_node->num = n; // Assigning data to the new node
new_node->next = head; // Pointing the new node to the current head
head = new_node; // Making the new node as the head
}
// Function to delete the first node of the list
void delete_first_node() {
if (head != NULL) { // Check if the list is not empty
Node* temp = head; // Temporary pointer to the current head
head = head->next; // Move the head to the next node
free(temp); // Free the memory of the original head node
}
}
// Function to display all nodes in the linked list
void display_all_nodes() {
struct Node *temp = head; // Temporary pointer to traverse the list
while (temp != NULL) { // Loop through all nodes until the end is reached
cout << temp->num << " "; // Displaying the data in the current node
temp = temp->next; // Move to the next node
}
}
int main() {
insert_Node(1); // Inserting a node with value 1
insert_Node(3); // Inserting a node with value 3
insert_Node(5); // Inserting a node with value 5
insert_Node(7); // Inserting a node with value 7
insert_Node(9); // Inserting a node with value 9
insert_Node(11); // Inserting a node with value 11
insert_Node(13); // Inserting a node with value 13
cout << "Original Linked list:\n"; // Displaying message for the original list
display_all_nodes(); // Displaying all nodes in the original list
cout << "\n\nDelete first node of Singly Linked List:\n"; // Displaying message for the deletion
delete_first_node(); // Deleting the first node of the list
display_all_nodes(); // Displaying all nodes after deletion
cout << endl; // Displaying newline
return 0; // Returning from the main function
}
Sample Output:
Original Linked list: 13 11 9 7 5 3 1 Delete first node of Singly Linked List: 11 9 7 5 3 1
Flowchart:
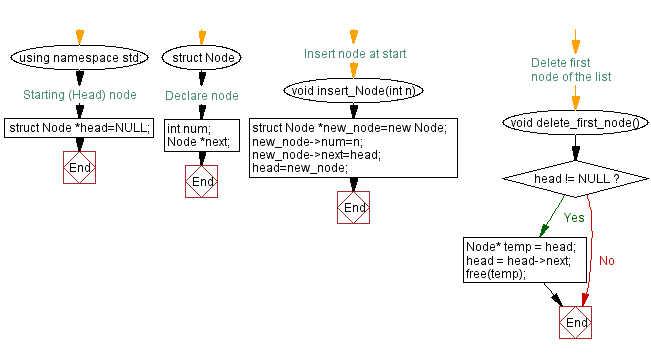
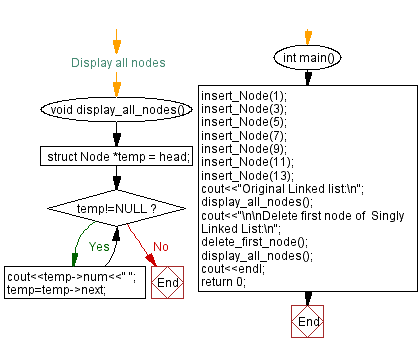
For more Practice: Solve these Related Problems:
- Write a C++ program to delete the first node of a singly linked list and then display the updated list.
- Develop a C++ program to remove the head node of a singly linked list and print both the removed value and the new head.
- Design a C++ program to delete the first node from a singly linked list while handling empty list scenarios.
- Implement a C++ program that deletes the first node of a singly linked list and confirms the deletion by checking the list length.
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Insert a node at any position of a Singly Linked List.
Next C++ Exercise: Delete a middle node from a Singly Linked List.
What is the difficulty level of this exercise?