C++ Exercises: Find the Greatest Common Divisor (GCD) of two numbers
Write a program in C++ to find the Greatest Common Divisor (GCD) of two numbers.
Visual Presentation:
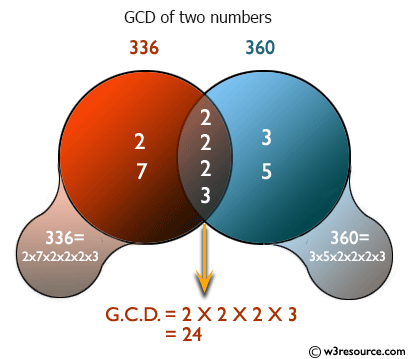
Sample Solution :-
C++ Code :
#include <iostream> // Preprocessor directive to include the input/output stream header file
using namespace std; // Using the standard namespace to avoid writing std::
int main() // Start of the main function
{
int num1, num2, gcd; // Declaration of integer variables 'num1', 'num2', and 'gcd'
// Display a message to find the Greatest Common Divisor (GCD) of two numbers
cout << "\n\n Find the Greatest Common Divisor of two numbers:\n";
cout << "-----------------------------------------------------\n";
// Prompt the user to input the first number
cout << " Input the first number: ";
cin >> num1; // Reading the first number entered by the user
// Prompt the user to input the second number
cout << " Input the second number: ";
cin >> num2; // Reading the second number entered by the user
// Loop to find the Greatest Common Divisor (GCD) of num1 and num2
for (int i = 1; i <= num1 && i <= num2; i++)
{
if (num1 % i == 0 && num2 % i == 0) // Check if 'i' divides both num1 and num2 evenly
{
gcd = i; // Update the 'gcd' variable with the current common divisor
}
}
// Display the Greatest Common Divisor (GCD) of the entered numbers
cout << " The Greatest Common Divisor is: " << gcd << endl;
return 0; // Indicating successful completion of the program
}
Sample Output:
Find the Greatest Common Divisor of two numbers: ----------------------------------------------------- Input the first number: 25 Input the second number: 15 The Greatest Common Divisor is: 5
Flowchart:
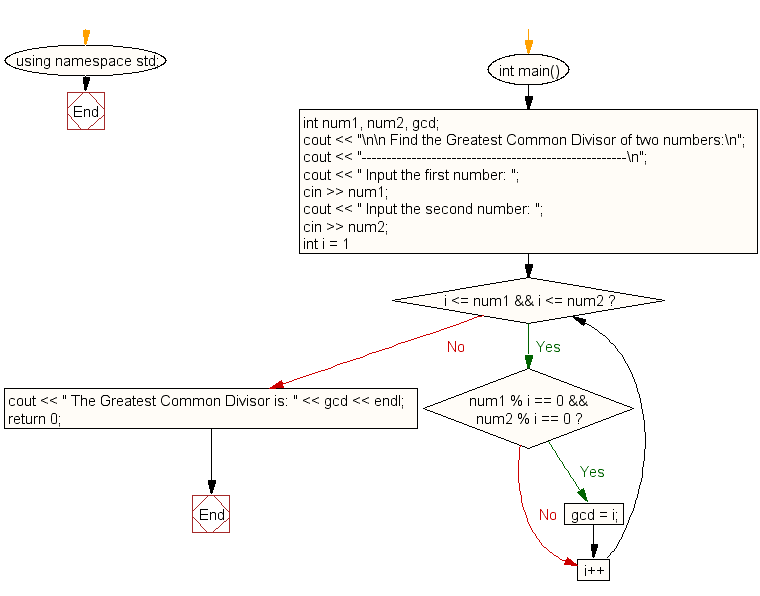
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to find the last prime number occur before the entered number.
Next: Write a program in C++ to find the sum of digits of a given number.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics