C++ Exercises: Create and display unique three-digit number using 1, 2, 3, 4
Write a C++ program to create and display an original three-digit numbers using 1, 2, 3, 4. Also count how many three-digit numbers are there.
Visual Presentation:
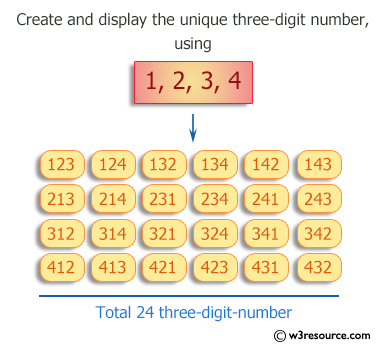
Sample Solution:-
C++ Code :
#include <iostream>
using namespace std;
// Function prototype declaration to reverse a string
void revOfString(const string& a);
int main() {
// Declaration of string variable to store output
string str;
// Displaying the purpose of the code
cout << "\n\n Create and display the unique three-digit number using 1, 2, 3, 4:\n";
cout << "-------------------------------------------------------------------\n";
// Displaying the prompt for three-digit numbers
cout << " The three-digit numbers are: " << endl;
// Initializing variable 'amount' to count the unique three-digit numbers
int amount = 0;
cout << " "; // Formatting output
// Loop to generate and display unique three-digit numbers
for (int i = 1; i <= 4; i++) {
for (int j = 1; j <= 4; j++) {
for (int k = 1; k <= 4; k++) {
// Checking for unique digits in each position (i, j, k)
if (k != i && k != j && i != j) {
amount++; // Incrementing the count of unique numbers
cout << i << j << k << " "; // Displaying the unique number
}
}
}
}
// Displaying the total count of unique three-digit numbers
cout << endl << " Total number of the three-digit numbers is: " << amount << endl << endl;
}
Sample Output:
Create and display the unique three-digit number using 1, 2, 3, 4: ------------------------------------------------------------------- The three-digit numbers are: 123 124 132 134 142 143 213 214 231 234 241 243 312 314 321 324 341 34 2 412 413 421 423 431 432 Total number of the three-digit-number is: 24
Flowchart:
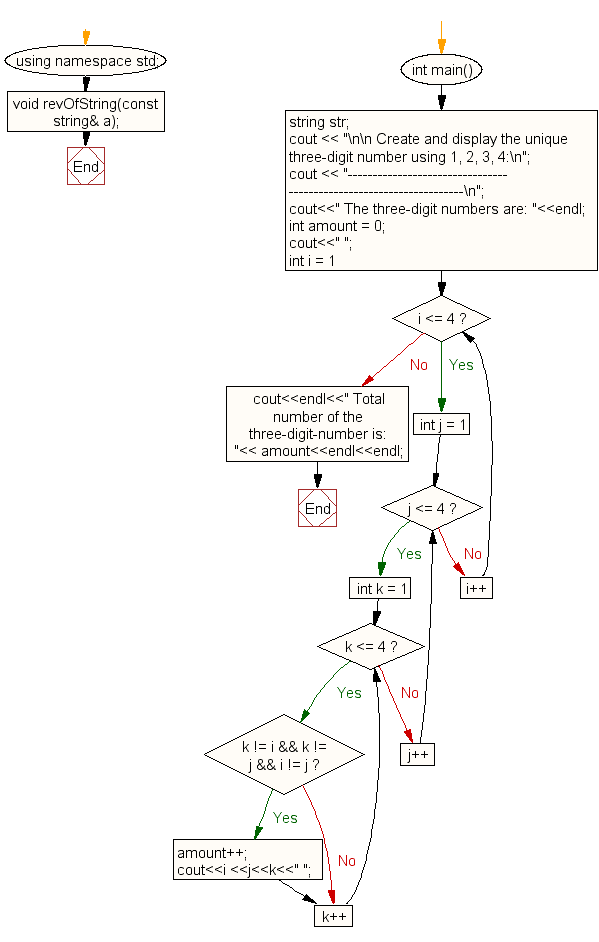
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to count the letters, spaces, numbers and other characters of an input string.
Next: C++ Array Exercises Home
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics