C++ Exercises: Count the letters, spaces, numbers and other characters of an input string.
Write a C++ program to count the letters, spaces, numbers and other characters in an input string.
Visual Presentation:
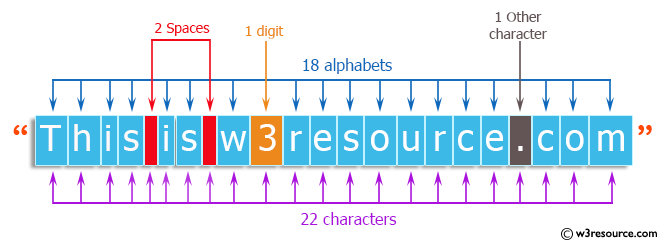
Sample Solution:-
C++ Code :
#include<iostream> // Header file for input-output stream
#include<string> // Header file for string handling
#include<cstring> // Header file for C-style string functions
using namespace std; // Using standard namespace
int main() // Main function
{
char *array_point; // Declaring a pointer to a character
char c1; // Declaring a character variable
int count = 0, alp = 0, digt = 0, spcchr = 0, oth = 0; // Initializing integer variables for counts
char string_array[100]; // Declaring an array of characters with size 100
string str1; // Declaring a string variable using C++ string class
// Displaying a message to count letters, spaces, numbers, and other characters
cout << "\n\n Count the letters, spaces, numbers and other characters:\n";
cout << "-------------------------------------------------------------\n";
cout << " Enter a string: ";
getline(cin, str1); // Taking the entire line as input and storing it in 'str1'
cout << endl;
strcpy(string_array, str1.c_str()); // Copying the string from str1 to string_array using strcpy
for (array_point = string_array; *array_point != '\0'; array_point++) // Looping through the string array
{
c1 = *array_point; // Assigning the character at array_point to c1
count++; // Incrementing the count of characters
if (isalpha(c1)) // Checking if the character is an alphabet
{
alp++; // Incrementing the count of alphabets
}
else if (isdigit(c1)) // Checking if the character is a digit
{
digt++; // Incrementing the count of digits
}
else if (isspace(c1)) // Checking if the character is a space
{
spcchr++; // Incrementing the count of spaces
}
else // If the character is neither alphabet, digit, nor space
{
oth++; // Incrementing the count of other characters
}
}
// Displaying the counts of different characters
cout << " The number of characters in the string is: " << count << endl;
cout << " The number of alphabets are: " << alp << endl;
cout << " The number of digits are: " << digt << endl;
cout << " The number of spaces are: " << spcchr << endl;
cout << " The number of other characters are: " << oth << endl << endl;
return 0; // Returning 0 to indicate successful execution
}
Sample Output:
Count the letters, spaces, numbers and other characters: ------------------------------------------------------------- Enter a string: This is w3resource.com The number of characters in the string is: 22 The number of alphabets are: 18 The number of digits are: 1 The number of spaces are: 2 The number of other characters are: 1
Flowchart:
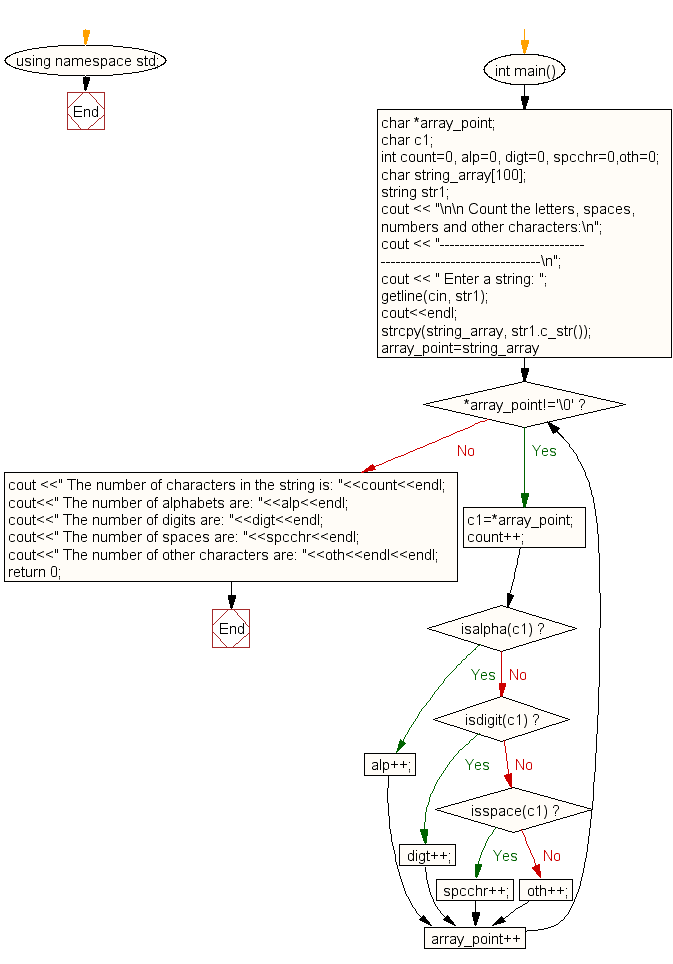
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to reverse a string.
Next: Write a program in C++ to create and display unique three-digit number using 1, 2, 3, 4. Also count how many three-digit numbers are there.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics