C++ Exercises: Reverse a string
85. Reverse a String
Write a program in C++ to reverse a string.
Visual Presentation:
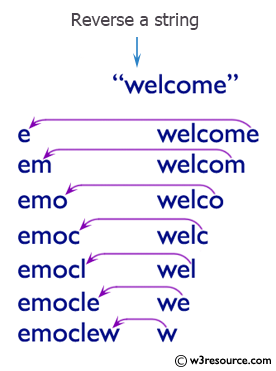
Sample Solution:-
C++ Code :
#include <iostream> // Including the input-output stream library
using namespace std; // Using standard namespace
// Function declaration to reverse the string recursively
void revOfString(const string& a);
int main() // Main function
{
string str; // Declaring a string variable 'str'
// Displaying a message to reverse a string
cout << "\n\n Reverse a string:\n";
cout << "----------------------\n";
// Asking the user to enter a string
cout << " Enter a string: ";
getline(cin, str); // Taking the entire line as input and storing it in 'str'
// Displaying a message before printing the reversed string
cout << " The string in reverse are: ";
// Calling the reverse string function
revOfString(str);
return 0; // Returning 0 to indicate successful execution
}
// Function definition to reverse the string recursively
void revOfString(const string& str)
{
size_t lengthOfString = str.size(); // Getting the length of the string
// Checking if the length of the string is 1, then simply print the character and end the line
if (lengthOfString == 1)
cout << str << endl;
else
{
// Print the last character of the string
cout << str[lengthOfString - 1];
// Recursively call the function by passing the substring from 0 to length - 1
revOfString(str.substr(0, lengthOfString - 1));
}
}
Sample Output:
Reverse a string: ---------------------- Enter a string: w3resource The string in reverse are: ecruoser3w
Flowchart:
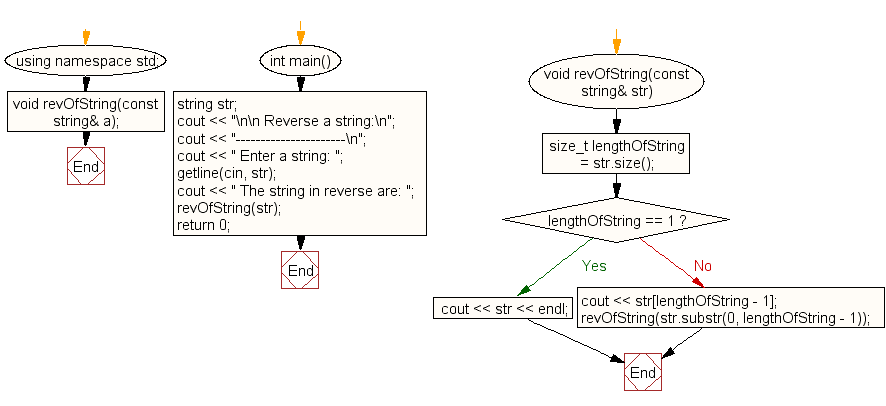
For more Practice: Solve these Related Problems:
- Write a C++ program to reverse an input string using a for loop and print the reversed string.
- Write a C++ program that reads a string and reverses it using recursion, then outputs the result.
- Write a C++ program to reverse a string by swapping characters from both ends using pointers.
- Write a C++ program to convert a string to a character array and then reverse the array to display the reversed string.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to compute the sum of the digits of an integer using function.
Next: Write a program in C++ to count the letters, spaces, numbers and other characters of an input string.
What is the difficulty level of this exercise?