C++ Exercises: Convert a hexadecimal number to decimal number
79. Hexadecimal to Decimal Conversion
Write a C++ program to convert a hexadecimal number to a decimal number.
Visual Presentation:
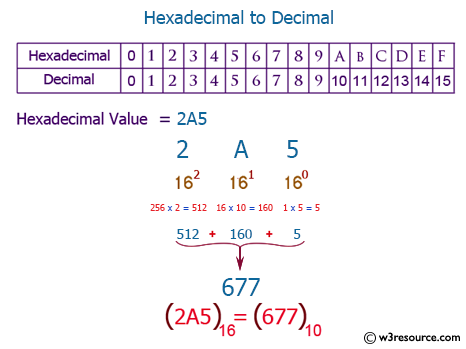
Sample Solution:-
C++ Code :
#include<iostream> // Include necessary libraries
#include<stdlib.h>
#include<math.h>
using namespace std; // Use the standard namespace
// Function to convert hexadecimal to decimal
unsigned long Hex_To_Dec(char hex[]) {
char *hexstring; // Pointer to the hexadecimal string
int length = 0; // Variable to store the length of the input string
const int hexbase = 16; // Constant to define base 16 for hexadecimal
unsigned long dnum = 0; // Variable to store the resulting decimal number
int i;
// Loop to calculate the length of the hexadecimal string
for (hexstring = hex; *hexstring != '\0'; hexstring++) {
length++;
}
hexstring = hex; // Reset the pointer to the start of the hexadecimal string
// Loop to convert each character of the hexadecimal string to decimal
for (i = 0; *hexstring != '\0' && i < length; i++, hexstring++) {
if (*hexstring >= 48 && *hexstring <= 57) {
// Convert character to decimal if it's a number (0-9)
dnum += (((int)(*hexstring)) - 48) * pow(hexbase, length - i - 1);
}
else if ((*hexstring >= 65 && *hexstring <= 70)) {
// Convert character to decimal if it's an uppercase letter (A-F)
dnum += (((int)(*hexstring)) - 55) * pow(hexbase, length - i - 1);
}
else if (*hexstring >= 97 && *hexstring <= 102) {
// Convert character to decimal if it's a lowercase letter (a-f)
dnum += (((int)(*hexstring)) - 87) * pow(hexbase, length - i - 1);
}
else {
// Display an error message for an invalid input character
cout << " The given hexadecimal number is invalid. \n";
}
}
return dnum; // Return the resulting decimal number
}
// Main function
int main() {
unsigned long dnum; // Variable to store the decimal number
char hex[9]; // Array to store the input hexadecimal number
cout << "\n\n Convert any hexadecimal number to decimal number:\n"; // Display purpose of the program
cout << "------------------------------------------------------\n"; // Display separator line
cout << " Input any 32-bit Hexadecimal Number: ";
cin >> hex; // Read the hexadecimal input from the user
dnum = Hex_To_Dec(hex); // Call the function to convert hexadecimal to decimal
cout << " The value in decimal number is: " << dnum << "\n"; // Display the resulting decimal number
}
Sample Output:
Convert any hexadecimal number to decimal number: ------------------------------------------------------ Input any 32-bit Hexadecimal Number: 25 The value in decimal number is: 37
Flowchart:
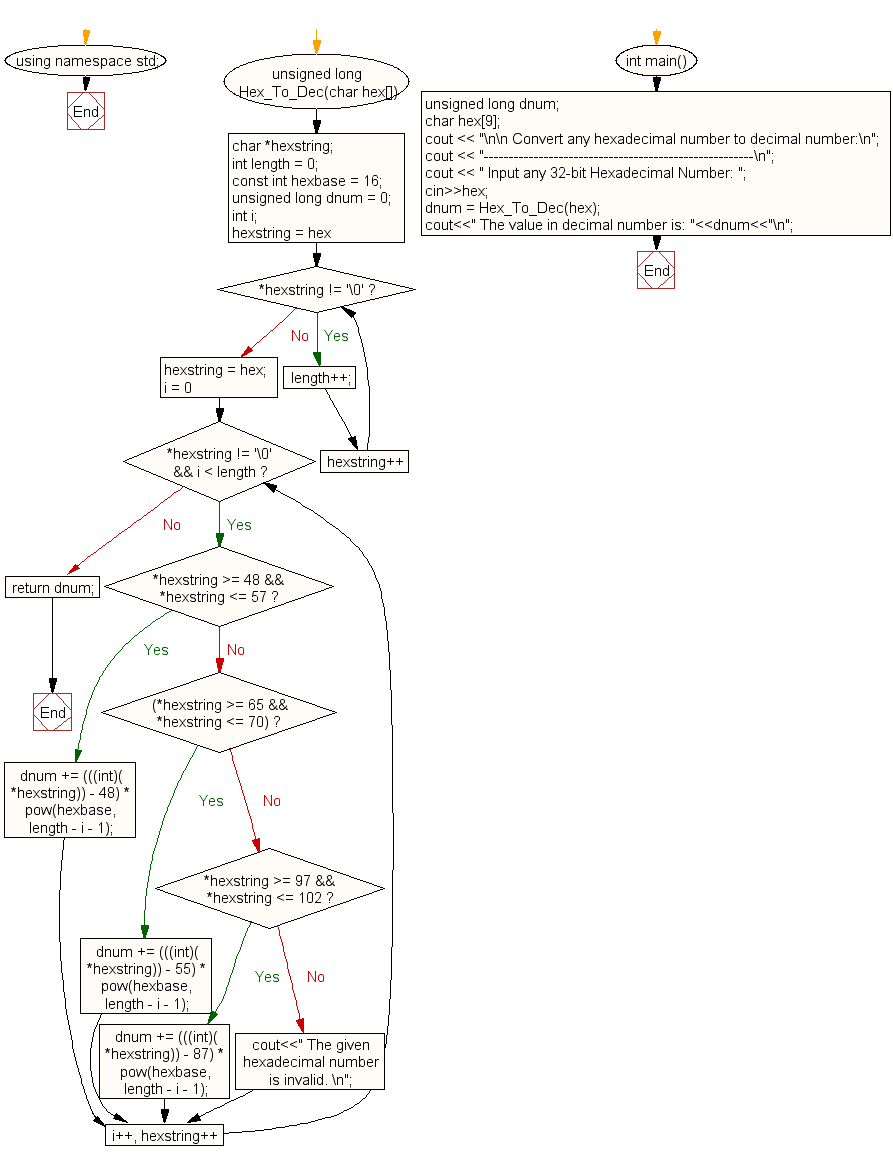
For more Practice: Solve these Related Problems:
- Write a C++ program to convert a hexadecimal number to its decimal equivalent by processing each hex digit.
- Write a C++ program that reads a hexadecimal string and calculates its decimal value using power functions.
- Write a C++ program to compute the decimal value of a 32-bit hexadecimal input by iterating over each character.
- Write a C++ program that converts a hexadecimal number to decimal using a loop and conditional mapping for digits A-F.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to convert a octal number to a hexadecimal number.
Next: Write a program in C++ to convert hexadecimal number to binary number.
What is the difficulty level of this exercise?