C++ Exercises: Convert a octal number to a hexadecimal number
78. Octal to Hexadecimal Conversion
Write a C++ program to convert an octal number to a hexadecimal number.
Visual Presentation:
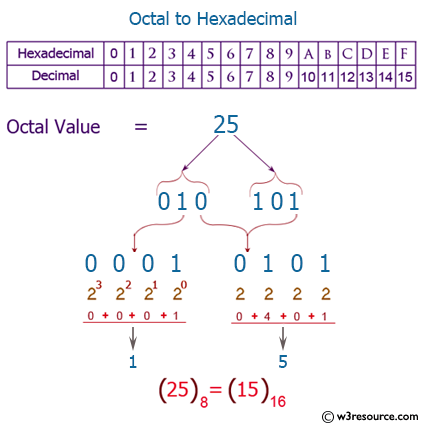
Sample Solution:-
C++ Code :
#include<iostream> // Include necessary libraries
#include<string.h>
#include<math.h>
using namespace std; // Use the standard namespace
int main() // Start of the main function
{
// Declare arrays and variables for calculations
int ar1[20], ar2[20], ar3[20];
int h, i, j, k, l, x, fr, flg, rem, n1, n3;
float rem1, n2, n4, dno;
char octal_num[20]; // Define a character array to store the octal number
x = fr = flg = rem = 0;
rem1 = 0.0;
cout << "\n\n Convert any octal number to a hexadecimal number:\n"; // Prompt the user for octal to hexadecimal conversion
cout << "------------------------------------------------------\n"; // Display separator line
cout << " Input any octal number: ";
cin >> octal_num; // Read the octal number input by the user
// Separate whole and fractional parts of the octal number
for (i = 0, j = 0, k = 0; i < strlen(octal_num); i++)
{
if (octal_num[i] == '.')
{
flg = 1;
}
else if (flg == 0)
ar1[j++] = octal_num[i] - 48;
else if (flg == 1)
ar2[k++] = octal_num[i] - 48;
}
x = j; // Store the count of whole part
fr = k; // Store the count of fractional part
// Convert whole part of octal to decimal
for (j = 0, i = x - 1; j < x; j++, i--)
{
rem = rem + (ar1[j] * pow(8, i));
}
// Convert fractional part of octal to decimal
for (k = 0, i = 1; k < fr; k++, i++)
{
rem1 = rem1 + (ar2[k] / pow(8, i));
}
rem1 = rem + rem1; // Combine the whole and fractional parts for calculation
dno = rem1; // Store the combined decimal number
n1 = (int)dno; // Extract the integer part
n2 = dno - n1; // Extract the fractional part
i = 0;
// Convert the integer part to hexadecimal
while (n1 != 0)
{
rem = n1 % 16;
ar3[i] = rem;
n1 = n1 / 16;
i++;
}
j = 0;
// Convert the fractional part to hexadecimal
while (n2 != 0.0)
{
n2 = n2 * 16;
n3 = (int)n2;
n4 = n2 - n3;
n2 = n4;
ar1[j] = n3;
j++;
if (j == 4)
{
break;
}
}
l = i; // Store the count of integer part
cout << " The hexadecimal value of " << octal_num << " is: ";
// Display the integer part in hexadecimal
for (i = l - 1; i >= 0; i--)
{
if (ar3[i] == 10)
cout << "A";
else if (ar3[i] == 11)
cout << "B";
else if (ar3[i] == 12)
cout << "C";
else if (ar3[i] == 13)
cout << "D";
else if (ar3[i] == 14)
cout << "E";
else if (ar3[i] == 15)
cout << "F";
else
cout << ar3[i];
}
h = j; // Store the count of fractional part
cout << ".";
// Display the fractional part in hexadecimal
for (k = 0; k < h; k++)
{
if (ar1[k] == 10)
cout << "A";
else if (ar1[k] == 11)
cout << "B";
else if (ar1[k] == 12)
cout << "C";
else if (ar1[k] == 13)
cout << "D";
else if (ar1[k] == 14)
cout << "E";
else if (ar1[k] == 15)
cout << "F";
else
cout << ar1[k];
}
cout << endl;
}
Sample Output:
Convert any octal number to a hexadecimal number: ------------------------------------------------------ Input any octal number: 77 The hexadecimal value of 77 is: 3F.
Flowchart:
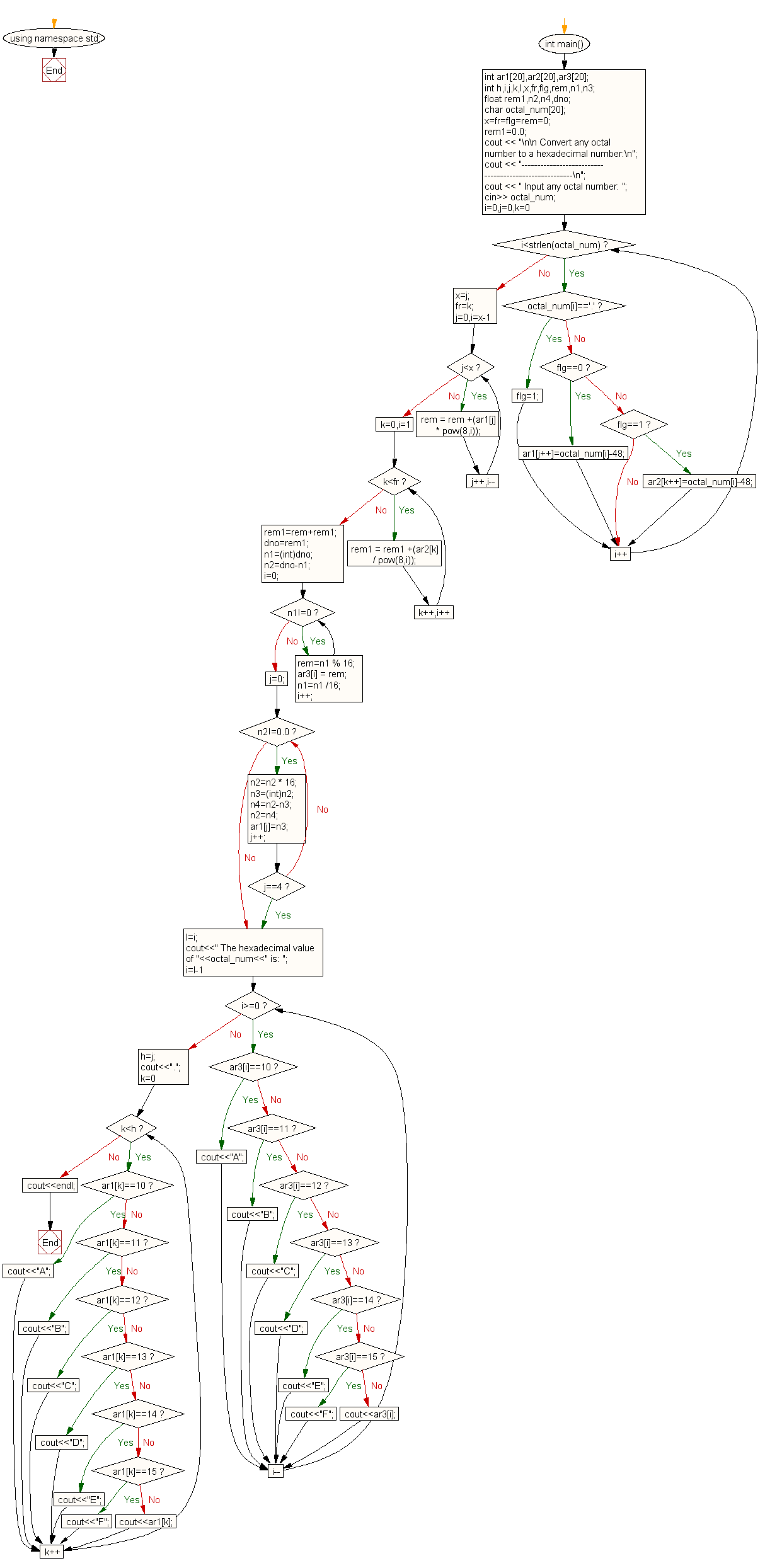
For more Practice: Solve these Related Problems:
- Write a C++ program to convert an octal number to hexadecimal by first converting it to decimal and then to hexadecimal.
- Write a C++ program that reads an octal input and outputs its hexadecimal equivalent using intermediate conversion.
- Write a C++ program to compute the hexadecimal representation of an octal number by mapping through its decimal value.
- Write a C++ program that converts an octal string to hexadecimal by chaining octal-to-decimal and decimal-to-hexadecimal conversions.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to convert a octal number to binary number.
Next: Write a program in C++ to convert a hexadecimal number to decimal number.
What is the difficulty level of this exercise?