C++ Exercises: Convert a octal number to decimal number
Write a C++ program to convert an octal number to a decimal number.
Visual Presentation:
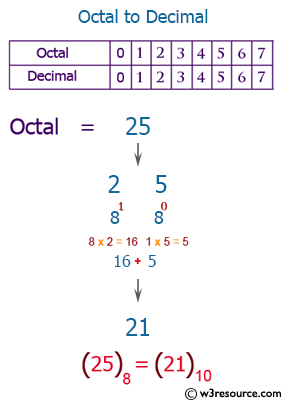
Sample Solution:-
C++ Code :
#include <iostream> // Include input/output stream library
#include <math.h> // Include math library for mathematical functions
using namespace std; // Use the standard namespace
int main() // Main function where the execution of the program starts
{
long octal_num, decimal_num = 0; // Declare variables for octal and decimal numbers
int i = 0; // Initialize an iteration variable
cout << "\n\n Convert any octal number to decimal number:\n"; // Display message prompting for octal to decimal conversion
cout << "----------------------------------------------------\n"; // Display separator line
cout << " Input any octal number: "; // Prompt user to input an octal number
cin >> octal_num; // Read the octal number input by the user
// Loop to convert octal to decimal
while (octal_num != 0)
{
decimal_num = (long)(decimal_num + (octal_num % 10) * pow(8, i++)); // Calculate the decimal number using powers of 8
octal_num = octal_num / 10; // Update the octal number by removing its last digit (rightmost digit)
}
cout << " The equivalent decimal number: " << decimal_num << "\n"; // Display the equivalent decimal number
}
Sample Output:
Convert any octal number to decimal number: ---------------------------------------------------- Input any octal number: 17 The equivalent decimal number: 15
Flowchart:
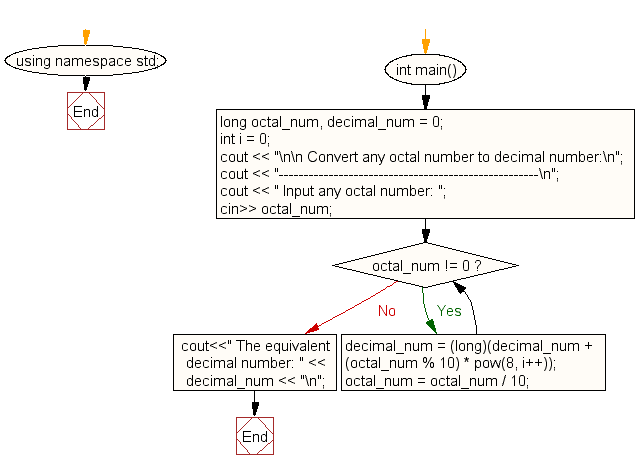
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to convert a binary number to octal number.
Next: Write a program in C++ to convert a octal number to binary number.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics