C++ Exercises: Convert a binary number to decimal number
73. Binary to Decimal Conversion
Write a C++ program to convert a binary number to a decimal number.
Visual Presentation:
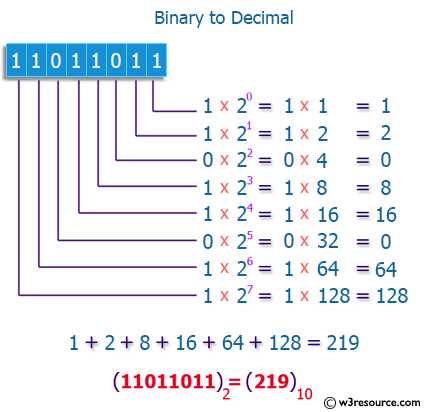
Sample Solution:-
C++ Code :
#include <iostream> // Include input/output stream library
#include <math.h> // Include math library for mathematical functions
using namespace std; // Use the standard namespace
int main() // Main function where the execution of the program starts
{
long binaryNumber, decimalNumber = 0, j = 1, remainder; // Declare variables for binary and decimal numbers, remainder, and a multiplier
cout << "\n\n Convert a binary number to decimal number:\n"; // Display message prompting for binary to decimal conversion
cout << "-----------------------------------------------\n"; // Display separator line
cout << " Input a binary number: "; // Prompt user to input a binary number
cin >> binaryNumber; // Read the binary number input by the user
while (binaryNumber != 0) // Loop to convert binary number to decimal
{
remainder = binaryNumber % 10; // Calculate the remainder by performing modulo 10 operation
decimalNumber = decimalNumber + remainder * j; // Calculate the decimal number by adding the remainder multiplied by a factor 'j'
j = j * 2; // Multiply the factor 'j' by 2 for the next iteration
binaryNumber = binaryNumber / 10; // Update the binary number by removing its last digit (rightmost digit)
}
cout << " The decimal number: " << decimalNumber << "\n"; // Display the resulting decimal number
}
Sample Output:
Convert a binary number to decimal number: ----------------------------------------------- Input a binary number: 1011 The decimal number: 11
Flowchart:
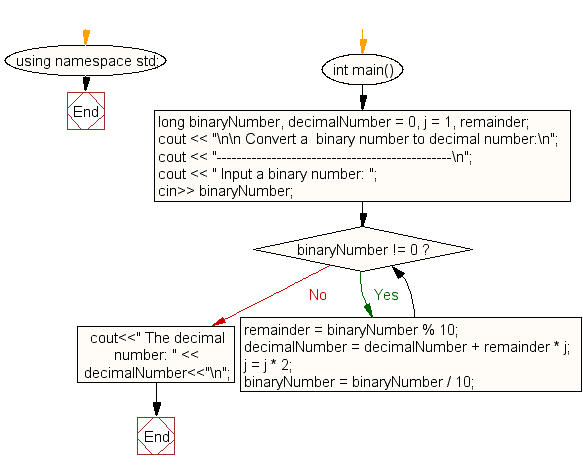
For more Practice: Solve these Related Problems:
- Write a C++ program to convert a binary number (as a string) to its decimal equivalent using iterative multiplication.
- Write a C++ program that reads an 8-bit binary number and calculates its decimal value using the power of 2.
- Write a C++ program to compute the decimal conversion of a binary input by processing each digit from right to left.
- Write a C++ program that accepts a binary string, converts it to decimal using a loop, and prints the result.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to convert a decimal number to octal number.
Next: Write a program in C++ to convert a binary number to hexadecimal number.
What is the difficulty level of this exercise?