C++ Exercises: Convert a decimal number to octal number
Write a C++ program to convert a decimal number to an octal number.
Visual Presentation:
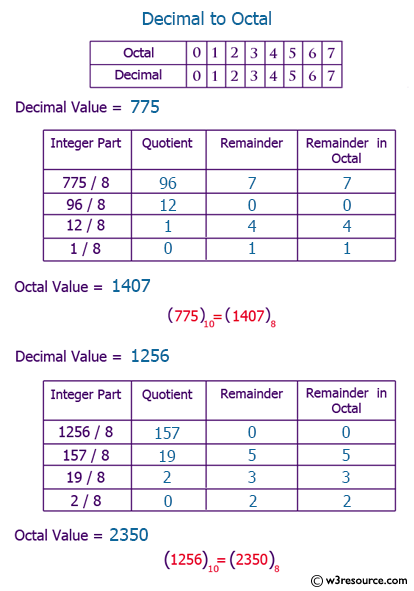
Sample Solution:-
C++ Code :
#include <iostream> // Include input/output stream library
#include <math.h> // Include math library for mathematical functions
using namespace std; // Use the standard namespace
int main() // Main function where the execution of the program starts
{
int dec_num, rem, quot, i = 1, j; // Declare integer variables for decimal number, remainder, quotient, and loop counters
int oct_num[100]; // Declare an array to store octal digits, initialized to store up to 100 digits
cout << "\n\n Convert a decimal number to octal number:\n"; // Display message prompting for decimal to octal conversion
cout << "-----------------------------------------------\n"; // Display separator line
cout << " Input a decimal number: "; // Prompt user to input a decimal number
cin >> dec_num; // Read the decimal number input by the user
quot = dec_num; // Assign the value of dec_num to quot (initializing quotient with the decimal number)
while (quot != 0) // Loop to convert decimal number to octal
{
oct_num[i++] = quot % 8; // Store remainder (quotient modulo 8) as octal digit in the array
quot = quot / 8; // Calculate next quotient by dividing it by 8 (octal base)
}
cout << " The octal number is: "; // Display message indicating the octal number
for (j = i - 1; j > 0; j--) // Loop to print octal digits in reverse order
{
cout << oct_num[j]; // Output octal digits from the array in reverse order
}
cout << "\n"; // Move to the next line after printing the octal number
}
Sample Output:
Convert a decimal number to octal number: ----------------------------------------------- Input a decimal number: 15 The octal number is: 17
Flowchart:
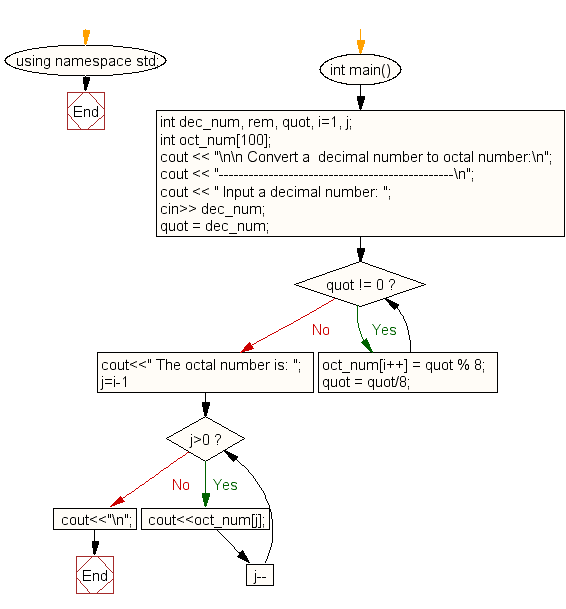
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to convert a decimal number to hexadecimal number.
Next: Write a program in C++ to convert a binary number to decimal number.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics