C++ Exercises: Convert a decimal number to hexadecimal number
Write a program in C++ to convert a decimal number to a hexadecimal number.
Visual Presentation:
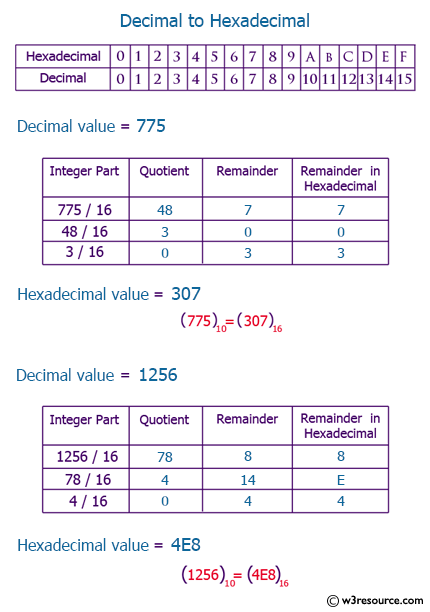
Sample Solution:-
C++ Code :
#include <iostream> // Include input/output stream library
#include <math.h> // Include math library for mathematical functions
using namespace std; // Use the standard namespace
int main() // Main function where the execution of the program starts
{
int dec_num, r; // Declare integer variables 'dec_num' for decimal number and 'r' for remainder
string hexdec_num=""; // Declare an empty string 'hexdec_num' to store the hexadecimal number
char hex[] = {'0','1','2','3','4','5','6','7','8','9','A','B','C','D','E','F'}; // Array 'hex' containing hexadecimal characters
cout << "\n\n Convert a decimal number to hexadecimal number:\n"; // Display message prompting for decimal to hexadecimal conversion
cout << "---------------------------------------------------\n"; // Display separator line
cout << " Input a decimal number: "; // Prompt user to input a decimal number
cin >> dec_num; // Read the decimal number input by the user
while (dec_num > 0) { // Loop to convert decimal number to hexadecimal
r = dec_num % 16; // Calculate the remainder when divided by 16 (hexadecimal base)
hexdec_num = hex[r] + hexdec_num; // Store the corresponding hexadecimal character in 'hexdec_num'
dec_num = dec_num / 16; // Update 'dec_num' by dividing it by 16 (hexadecimal conversion process)
}
cout << " The hexadecimal number is : " << hexdec_num << "\n"; // Display the converted hexadecimal number
}
Sample Output:
Convert a decimal number to hexadecimal number: --------------------------------------------------- Input a decimal number: 43 The hexadecimal number is : 2B
Flowchart:
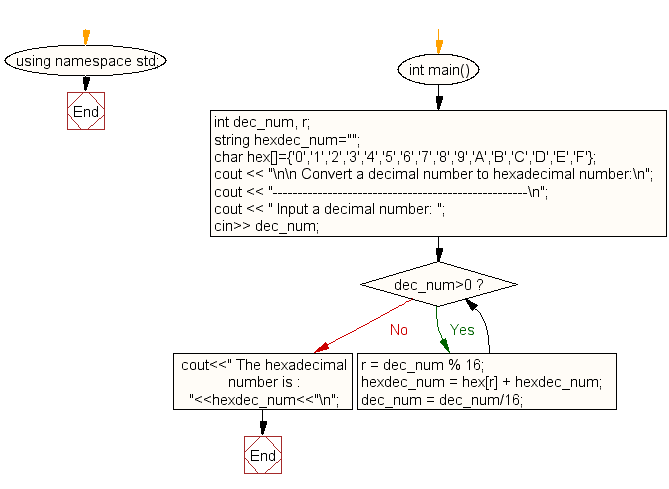
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to convert a decimal number to binary number.
Next: Write a program in C++ to convert a decimal number to octal number.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics