C++ Exercises: Convert a decimal number to binary number
70. Decimal to Binary Conversion
Write a program in C++ to convert a decimal number to a binary number.
Visual Presentation:
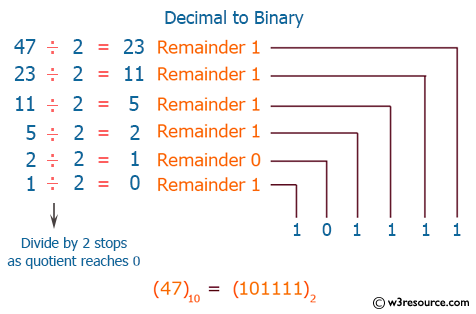
Sample Solution:-
C++ Code :
#include <iostream> // Include input/output stream library
#include <math.h> // Include math library for mathematical functions
using namespace std; // Use the standard namespace
int main() // Main function where the execution of the program starts
{
int dec_num, rem, quot, i = 1, j; // Declare integer variables 'dec_num', 'rem', 'quot', 'i', 'j' and initialize 'i' as 1
int bin_num[100]; // Declare an integer array 'bin_num' of size 100 to store binary digits
cout << "\n\n Convert a decimal number to binary number:\n"; // Display message prompting for decimal to binary conversion
cout << "---------------------------------------------------\n"; // Display separator line
cout << " Input a decimal number: "; // Prompt user to input a decimal number
cin >> dec_num; // Read the decimal number input by the user
quot = dec_num; // Assign the decimal number to 'quot' initially
while (quot != 0) { // Loop to convert decimal number to binary
bin_num[i++] = quot % 2; // Store remainder of division by 2 (binary digit) in 'bin_num' array and increment 'i'
quot = quot / 2; // Update 'quot' by dividing it by 2 (binary conversion process)
}
cout << " The binary number is: "; // Display message indicating the binary number output
for (j = i - 1; j > 0; j--) { // Loop to display the binary number stored in 'bin_num' array in reverse order
cout << bin_num[j]; // Output each binary digit from 'bin_num' array
}
cout << "\n"; // Move to the next line after displaying the binary number
}
Sample Output:
Convert a decimal number to binary number: --------------------------------------------------- Input a decimal number: 35 The binary number is: 100011
Flowchart:
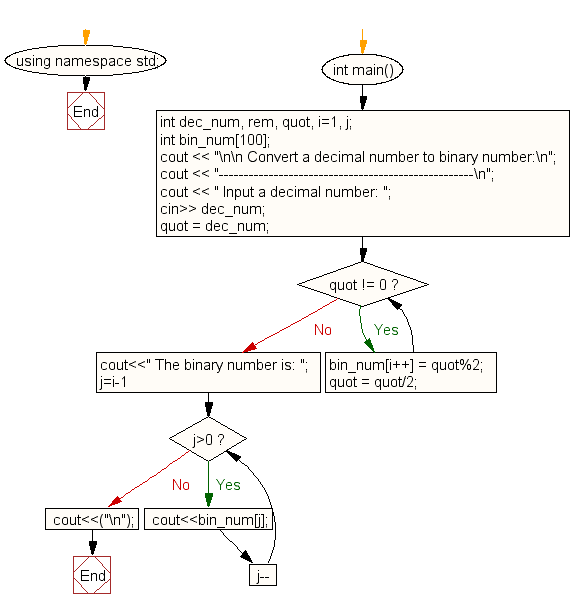
For more Practice: Solve these Related Problems:
- Write a C++ program to convert a decimal number to its binary representation using iterative division by 2.
- Write a C++ program that reads a decimal integer and outputs the corresponding binary number as a string.
- Write a C++ program to compute the binary equivalent of a decimal number using recursion and print the result.
- Write a C++ program that converts a decimal number to binary by repeatedly taking remainders and assembling the result in reverse order.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to produce a square matrix with 0's down the main diagonal, 1's in the
entries just above and below the main diagonal, 2's above and below that, etc.
Next: Write a program in C++ to convert a decimal number to hexadecimal number.
What is the difficulty level of this exercise?